EN
Node.js - PostgreSQL - find duplicated values in multiple columns
0
points
In this article, we would like to show you how to find duplicated valuesΒ in multiple columns in the PostgreSQL database usingΒ Node.js.
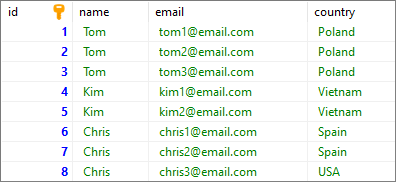
Note:Β at the end of this article you can find database preparation SQL queries.
const { Client } = require('pg');
const client = new Client({
host: '127.0.0.1',
user: 'postgres',
database: 'database_name',
password: 'password',
port: 5432,
});
const fetchDuplicateCollumns = async () => {
const query = `
SELECT
"name", COUNT("name") AS "name_count",
"country", COUNT("country") AS "country_count"
FROM "users"
GROUP BY "name", "country"
HAVING (COUNT("name") > 1) AND (COUNT("country") > 1);`;
try {
await client.connect(); // creates connection
const { rows } = await client.query(query); // sends query
console.table(rows);
} catch (error) {
console.error(error.stack);
} finally {
await client.end(); // closes connection
}
};
fetchDuplicateCollumns();
Result:Β
βββββββββββ¬ββββββββββ¬βββββββββββββ¬ββββββββββββ¬ββββββββββββββββ
β (index) β name β name_count β country β country_count β
βββββββββββΌββββββββββΌβββββββββββββΌββββββββββββΌββββββββββββββββ€
β 0 β 'Kim' β '2' β 'Vietnam' β '2' β
β 1 β 'Chris' β '2' β 'Spain' β '2' β
β 2 β 'Tom' β '3' β 'Poland' β '3' β
βββββββββββ΄ββββββββββ΄βββββββββββββ΄ββββββββββββ΄ββββββββββββββββ
Note:
Notice that
Chris
Β user bothΒΒ and
name_count
Β equals
country_count
2
because one user is from a different country.
Database preparation
create_tables.sql
Β file:
CREATE TABLE "users" (
"id" SERIAL,
"name" VARCHAR(100) NOT NULL,
"email" VARCHAR(100) NOT NULL,
"country" VARCHAR(15) NOT NULL,
PRIMARY KEY ("id")
);
insert_data.sql
Β file:
INSERT INTO "users"
("name", "email", "country")
VALUES
('Tom', 'tom1@email.com', 'Poland'),
('Tom', 'tom2@email.com', 'Poland'),
('Tom', 'tom3@email.com', 'Poland'),
('Kim', 'kim1@email.com', 'Vietnam'),
('Kim', 'kim2@email.com', 'Vietnam'),
('Chris', 'chris1@email.com', 'Spain'),
('Chris', 'chris2@email.com', 'Spain'),
('Chris', 'chris3@email.com', 'USA');
Native SQL query (used in the above example):
SELECT "name", COUNT("name") AS "name_count"
FROM "users"
GROUP BY "name"
HAVING COUNT("name") > 1