EN
JavaScript - copy text to system clipboard
15 points
In this article, we would like to show how to copy some text into the system clipboard in JavaScript.
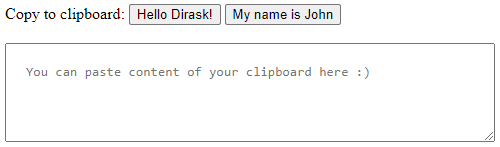
Quick solution:
xxxxxxxxxx
1
navigator.clipboard.writeText('Hello Dirask!'); // WORKS ONLY IN MODERN BROWSERS !!!
2
3
// go to the next section to custom example to see the universal solution.
The article shows two approaches:
- with modern JavaScript API - that approach may not be supported by some browsers,
- with a custom class that uses some trick to copy text into the system clipboard.
This API has working draft status and is not supported by all web browsers.
More information about the API is available here.
xxxxxxxxxx
1
2
<html>
3
<body>
4
<div>
5
<span>Copy to clipboard:</span>
6
<button onclick="navigator.clipboard.writeText('Hello Dirask!')">Hello Dirask!</button>
7
<button onclick="navigator.clipboard.writeText('My name is John')">My name is John</button>
8
</div>
9
<br />
10
<textarea style="padding: 20px; width: 300px;" placeholder="You can paste content of your clipboard here :)"></textarea>
11
</body>
12
</html>
The example presented in this section is universal and works in each browser. To understand how class works read the note below example.
xxxxxxxxxx
1
2
<html>
3
<head>
4
<script>
5
6
window.Clipboard = new function() {
7
var transit = null;
8
var element = null;
9
function copy(text) {
10
element.value = text;
11
element.style.display = 'block';
12
element.select();
13
document.execCommand('copy');
14
element.style.display = 'none';
15
}
16
window.addEventListener('load', function() {
17
element = document.createElement('textarea');
18
element.setAttribute('style', 'border: none; width: 0; height: 0; display: none');
19
document.body.appendChild(element);
20
if (transit) {
21
copy(transit);
22
}
23
});
24
this.copy = function(text) {
25
if (element) {
26
if (element.parentNode == null) {
27
throw new Error('Element has been removed from document.');
28
}
29
copy(text);
30
} else {
31
transit = text;
32
}
33
};
34
};
35
36
// Usage example:
37
//
38
// Clipboard.copy('Hello Dirask!')
39
40
</script>
41
</head>
42
<body>
43
<div>
44
<span>Copy to clipboard:</span>
45
<button onclick="Clipboard.copy('Hello Dirask!')">Hello Dirask!</button>
46
<button onclick="Clipboard.copy('My name is John')">My name is John</button>
47
</div>
48
<br />
49
<textarea style="padding: 20px; width: 300px;" placeholder="You can paste content of your clipboard here :)"></textarea>
50
</body>
51
</html>
Explanation:
textarea
supports multiline texts,textarea
element is set to0x0
px
to make it invisible for user during copy operation - to element prevent flickering on the web site,- to make text copy some simple tricks are necessary:
- text should be put inside text field,
- it is possible to make text copy only if text field is visible - because of security reasons - this is why element visibility is changed to visible during the operation,
- to make copy it is necessary to select copied text before the operation
- copy operation is realised by
copy
command.
Note: after click on the buttons you can paste text to some plase.