EN
JavaScript - touch events example (on touch screen)
5
points
In this article, we would like to show how to use touch events on touch screen under JavaScript.
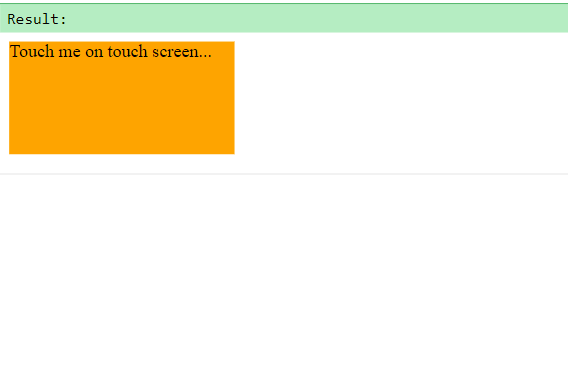
Quick solutions:
1. HTML event attribute:
<div ontouchstart="myFunction(event)"></div>
<!-- add more events: ontouchmove, ontouchend and ontouchcancel -->
<script>
function myFunction(e) {
// Source code here ...
}
</script>
2. Element event property
<div id="element"></div>
<script>
var element = document.querySelector('#element');
element.ontouchstart = function(e) { /* Source code here ... */ };
// add more events: ontouchmove, ontouchend and ontouchcancel
</script>
3. Element event listener
<div id="element"></div>
<script>
var element = document.querySelector('#element');
element.addEventListener('touchstart',Ā function(e) { /* Source code here ... */ });
// add more events: touchmove, touchend and touchcancel
</script>
Ā
There are available following events:
Event name | Event description |
---|---|
touchstart |
Occurs when on touch screen someĀ touch pointĀ appears. |
touchmove |
Occurs when some touch point changes position. |
touchend | Occurs when on touch screen someĀ touch point is removed. e.g. user takes someĀ fingerĀ from the screen. |
touchcancel | Occurs when some touch point case is not supported. e.g. too many touch points are used. |
Warning: the events will be not working with mouse.
Ā
Practical examples
In this section, we have placed practical exmaples for the quick solutions.
1. HTML event attribute example
// ONLINE-RUNNER:browser;
<!doctype html>
<html>
<head>
<style>
div.element {
background: orange;
width: 200px;
height: 100px;
}
</style>
</head>
<body>
<div
class="element"
ontouchstart="handleOnTouchStart(event)"
ontouchmove="handleOnTouchMove(event)"
ontouchend="handleOnTouchEnd(event)"
ontouchcancel="handleOnTouchCancel(event)"
>
Touch me on touch screen...
</div>
<script>
function handleOnTouchStart(e) {
var points = e.touches;
console.log('Element touched! touch points: ' + getPoints(points));
}
function handleOnTouchMove(e) {
var points = e.touches;
console.log('Element touched! touch points: ' + getPoints(points));
}
function handleOnTouchEnd(e) {
var points = e.touches;
console.log('Element touched! touch points: ' + getPoints(points));
}
function handleOnTouchCancel(e) {
var points = e.touches;
console.log('Element touched! touch points: ' + getPoints(points));
}
// Utils:
function getPoints(points) {
if (points.length === 0) {
return '<none>';
}
var result = '';
for (let i = 0; i < points.length; ++i) {
var point = points[i];
if (i > 0) {
result += ', ';
}
result += '(' + point.clientX + ', ' + point.clientY + ')';
}
return result;
}
</script>
</body>
</html>
Ā
2.Ā Element event property example
// ONLINE-RUNNER:browser;
<!doctype html>
<html>
<head>
<style>
div.element {
background: orange;
width: 200px;
height: 100px;
}
</style>
</head>
<body>
<div id="element" class="element">Touch me on touch screen...</div>
<script>
var element = document.querySelector('#element');
element.ontouchstart = function(e) {
var points = e.touches;
console.log('Element touched! (touch points: ' + points.length + ')');
};
element.ontouchmove = function(e) {
var points = e.touches;
console.log('Touch moved! (touch points: ' + points.length + ')');
};
element.ontouchend = function(e) {
var points = e.touches;
console.log('Touch ended! (touch points: ' + points.length + ')');
};
element.ontouchcancel = function(e) {
var points = e.touches;
console.log('Touch canceled! (touch points: ' + points.length + ')');
};
</script>
</body>
</html>
Ā
3. Element event listener example
// ONLINE-RUNNER:browser;
<!doctype html>
<html>
<head>
<style>
div.element {
background: orange;
width: 200px;
height: 100px;
}
</style>
</head>
<body>
<div id="element" class="element">Touch me on touch screen...</div>
<script>
var element = document.querySelector('#element');
element.addEventListener('touchstart', function(e) {
var points = e.touches;
console.log('Element touched! (touch points: ' + points.length + ')');
});
element.addEventListener('touchmove', function(e) {
var points = e.touches;
console.log('Touch moved! (touch points: ' + points.length + ')');
});
element.addEventListener('touchend', function(e) {
var points = e.touches;
console.log('Touch ended! (touch points: ' + points.length + ')');
});
element.addEventListener('touchcancel', function(e) {
var points = e.touches;
console.log('Touch canceled! (touch points: ' + points.length + ')');
});
</script>
</body>
</html>
Ā