EN
JavaScript - touch events example (on touch screen)
5 points
In this article, we would like to show how to use touch events on touch screen under JavaScript.
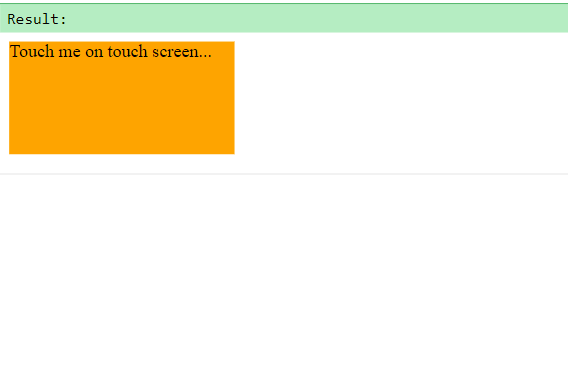
Quick solutions:
1. HTML event attribute:
xxxxxxxxxx
1
<div ontouchstart="myFunction(event)"></div>
2
<!-- add more events: ontouchmove, ontouchend and ontouchcancel -->
3
<script>
4
5
function myFunction(e) {
6
// Source code here ...
7
}
8
9
</script>
2. Element event property
xxxxxxxxxx
1
<div id="element"></div>
2
<script>
3
4
var element = document.querySelector('#element');
5
6
element.ontouchstart = function(e) { /* Source code here ... */ };
7
8
// add more events: ontouchmove, ontouchend and ontouchcancel
9
10
</script>
3. Element event listener
xxxxxxxxxx
1
<div id="element"></div>
2
<script>
3
4
var element = document.querySelector('#element');
5
6
element.addEventListener('touchstart', function(e) { /* Source code here ... */ });
7
8
// add more events: touchmove, touchend and touchcancel
9
10
</script>
There are available following events:
Event name | Event description |
---|---|
touchstart |
Occurs when on touch screen some touch point appears. |
touchmove |
Occurs when some touch point changes position. |
touchend | Occurs when on touch screen some touch point is removed. e.g. user takes some finger from the screen. |
touchcancel | Occurs when some touch point case is not supported. e.g. too many touch points are used. |
Warning: the events will be not working with mouse.
In this section, we have placed practical exmaples for the quick solutions.
xxxxxxxxxx
1
2
<html>
3
<head>
4
<style>
5
6
div.element {
7
background: orange;
8
width: 200px;
9
height: 100px;
10
}
11
12
</style>
13
</head>
14
<body>
15
<div
16
class="element"
17
ontouchstart="handleOnTouchStart(event)"
18
ontouchmove="handleOnTouchMove(event)"
19
ontouchend="handleOnTouchEnd(event)"
20
ontouchcancel="handleOnTouchCancel(event)"
21
>
22
Touch me on touch screen...
23
</div>
24
<script>
25
26
function handleOnTouchStart(e) {
27
var points = e.touches;
28
console.log('Element touched! touch points: ' + getPoints(points));
29
}
30
function handleOnTouchMove(e) {
31
var points = e.touches;
32
console.log('Element touched! touch points: ' + getPoints(points));
33
}
34
function handleOnTouchEnd(e) {
35
var points = e.touches;
36
console.log('Element touched! touch points: ' + getPoints(points));
37
}
38
function handleOnTouchCancel(e) {
39
var points = e.touches;
40
console.log('Element touched! touch points: ' + getPoints(points));
41
}
42
43
44
// Utils:
45
46
function getPoints(points) {
47
if (points.length === 0) {
48
return '<none>';
49
}
50
var result = '';
51
for (let i = 0; i < points.length; ++i) {
52
var point = points[i];
53
if (i > 0) {
54
result += ', ';
55
}
56
result += '(' + point.clientX + ', ' + point.clientY + ')';
57
}
58
return result;
59
}
60
61
</script>
62
</body>
63
</html>
xxxxxxxxxx
1
2
<html>
3
<head>
4
<style>
5
6
div.element {
7
background: orange;
8
width: 200px;
9
height: 100px;
10
}
11
12
</style>
13
</head>
14
<body>
15
<div id="element" class="element">Touch me on touch screen...</div>
16
<script>
17
18
var element = document.querySelector('#element');
19
20
element.ontouchstart = function(e) {
21
var points = e.touches;
22
console.log('Element touched! (touch points: ' + points.length + ')');
23
};
24
element.ontouchmove = function(e) {
25
var points = e.touches;
26
console.log('Touch moved! (touch points: ' + points.length + ')');
27
};
28
element.ontouchend = function(e) {
29
var points = e.touches;
30
console.log('Touch ended! (touch points: ' + points.length + ')');
31
};
32
element.ontouchcancel = function(e) {
33
var points = e.touches;
34
console.log('Touch canceled! (touch points: ' + points.length + ')');
35
};
36
37
</script>
38
</body>
39
</html>
xxxxxxxxxx
1
2
<html>
3
<head>
4
<style>
5
6
div.element {
7
background: orange;
8
width: 200px;
9
height: 100px;
10
}
11
12
</style>
13
</head>
14
<body>
15
<div id="element" class="element">Touch me on touch screen...</div>
16
<script>
17
18
var element = document.querySelector('#element');
19
20
element.addEventListener('touchstart', function(e) {
21
var points = e.touches;
22
console.log('Element touched! (touch points: ' + points.length + ')');
23
});
24
25
element.addEventListener('touchmove', function(e) {
26
var points = e.touches;
27
console.log('Touch moved! (touch points: ' + points.length + ')');
28
});
29
30
element.addEventListener('touchend', function(e) {
31
var points = e.touches;
32
console.log('Touch ended! (touch points: ' + points.length + ')');
33
});
34
35
element.addEventListener('touchcancel', function(e) {
36
var points = e.touches;
37
console.log('Touch canceled! (touch points: ' + points.length + ')');
38
});
39
40
</script>
41
</body>
42
</html>