EN
JavaScript - simple way how to write own function to make beauty string shortcuts
7 points
In this article, we're going to have a look at how to write in JavaScript own function that creates shortcuts for string.
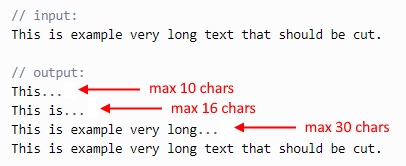
There are available styles to achieve the same effect, but sometimes we are not able to do it with styles and we need to have shortcut string e.g canvas
string drawing or title
/ alt
attribute.
This approach solves somehow the problem of multiline text with ...
in the end, if we don't want to display all text because CSS solutions are not enough at this moment (we have 2020).
The presented solution in this section tries to find closes white characters to cut the string in a pretty way - to do not split worlds. Only when it is impossible to find the white characters, function cuts worlds.
xxxxxxxxxx
1
var Utils = new function() {
2
var expression = /\s[^\s]*$/;
3
this.createShortcut = function(text, limit) {
4
if (text.length > limit) {
5
var part = text.slice(0, limit - 3);
6
if (part.match(expression)) {
7
return part.replace(expression, '...');
8
}
9
return part + '...';
10
}
11
return text;
12
};
13
};
14
15
// Usage example:
16
17
// number: |10 |16 |30
18
// v v v
19
var text = 'This is example very long text that should be cut.';
20
// cutting: | | |
21
22
console.log(Utils.createShortcut(text, 10)); // This...
23
console.log(Utils.createShortcut(text, 16)); // This is...
24
console.log(Utils.createShortcut(text, 30)); // This is example very long...
25
console.log(Utils.createShortcut(text, 250)); // This is example very long text that should be cut.
26
27
// number: |10 |16 |30
28
// v v v
29
var text = 'This_is_example_very_long_text_that_should_be_cut.';
30
// cutting: | | |
31
32
console.log(Utils.createShortcut(text, 10)); // This_is...
33
console.log(Utils.createShortcut(text, 16)); // This_is_examp...
34
console.log(Utils.createShortcut(text, 30)); // This_is_example_very_long_t...
35
console.log(Utils.createShortcut(text, 250)); // This is example very long text that should be cut.
xxxxxxxxxx
1
const expression = /\s[^\s]*$/;
2
3
const createShortcut = (text, limit) => {
4
if (text.length > limit) {
5
const part = text.slice(0, limit - 3);
6
if (part.match(expression)) {
7
return part.replace(expression, '...');
8
}
9
return part + '...';
10
}
11
return text;
12
};
13
14
15
// Usage example:
16
17
// number: |10 |16 |30
18
// v v v
19
const text1 = 'This is example very long text that should be cut.';
20
// cutting: | | |
21
22
console.log(createShortcut(text1, 10)); // This...
23
console.log(createShortcut(text1, 16)); // This is...
24
console.log(createShortcut(text1, 30)); // This is example very long...
25
console.log(createShortcut(text1, 250)); // This is example very long text that should be cut.
26
27
// number: |10 |16 |30
28
// v v v
29
const text2 = 'This_is_example_very_long_text_that_should_be_cut.';
30
// cutting: | | |
31
32
console.log(createShortcut(text2, 10)); // This_is...
33
console.log(createShortcut(text2, 16)); // This_is_examp...
34
console.log(createShortcut(text2, 30)); // This_is_example_very_long_t...
35
console.log(createShortcut(text2, 250)); // This is example very long text that should be cut.
xxxxxxxxxx
1
const isWhiteCharacter = (value) => value === ' ' || value === '\f' || value === '\n' || value === '\r' || value === '\t' || value === '\v' || value === '\u00a0' || value === '\u1680' || value === '\u2000' || value === '\u2001' || value === '\u2002' || value === '\u2003' || value === '\u2004' || value === '\u2005' || value === '\u2006' || value === '\u2007' || value === '\u2008' || value === '\u2009' || value === '\u200a' || value === '\u2028' || value === '\u2029' || value === '\u202f' || value === '\u205f' || value === '\u3000' || value === '\ufeff';
2
3
const createShortcut = (text, limit) => {
4
if (text.length > limit) {
5
let ending = false;
6
for (let i = limit - 3; i > -1; --i) {
7
if (isWhiteCharacter(text[i])) {
8
ending = true;
9
} else {
10
if (ending) {
11
limit = i + 4;
12
break;
13
}
14
}
15
}
16
const part = text.substring(0, limit - 3);
17
return part + '...';
18
}
19
return text;
20
};
21
22
23
// Usage example:
24
25
// number: |9 |16 |30
26
// v v v
27
const text1 = 'This is example very long text that should be cut.';
28
// cutting: | | |
29
30
console.log(createShortcut(text1, 9)); // This...
31
console.log(createShortcut(text1, 16)); // This is...
32
console.log(createShortcut(text1, 30)); // This is example very long...
33
console.log(createShortcut(text1, 250)); // This is example very long text that should be cut.
34
35
// number: |9 |16 |30
36
// v v v
37
const text2 = 'This_is_example_very_long_text_that_should_be_cut.';
38
// cutting: | | |
39
40
console.log(createShortcut(text2, 9)); // This_i...
41
console.log(createShortcut(text2, 16)); // This_is_examp...
42
console.log(createShortcut(text2, 30)); // This_is_example_very_long_t...
43
console.log(createShortcut(text2, 250)); // This_is_example_very_long_text_that_should_be_cut.