EN
JavaScript - create HTML shortcut
9
points
In this short article, we would like to show how to create shortcut for indicated HTML using JavaScript.
As HTML shortcut we understand inner text and HTML elements cut to desired length.
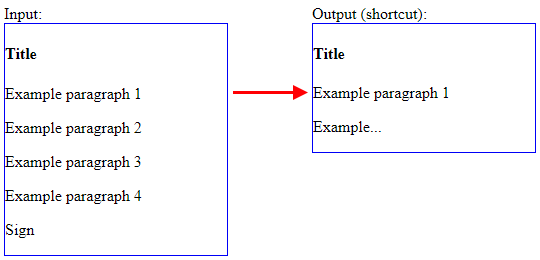
Practical example:
// ONLINE-RUNNER:browser;
<!doctype html>
<html>
<head>
<style>
div.html {
border: 1px solid blue;
}
div.shortcut {
max-height: 150px;
text-overflow: ellipsis;
white-space: nowrap;
overflow: hidden;
}
div.section + div.section {
margin: 20px 0 0 0;
}
</style>
</head>
<body>
<div class="section">
<div class="title">Input:</div>
<div class="html input" id="input">
<h4>Title</h4>
<p>Example paragraph 1</p>
<p>Example paragraph 2</p>
<p>Example paragraph 3</p>
<p>Example paragraph 4</p>
<p>Sign</p>
</div>
</div>
<div class="section">
<div class="title">Output (shortcut):</div>
<div class="html shortcut" id="shortcut"></div>
</div>
<script>
function createArray(collection) {
var result = new Array(collection.length);
for (var i = 0; i < collection.length; ++i) {
result[i] = collection[i];
}
return result;
}
function createTemplate(html) {
var element = document.createElement('template');
element.innerHTML = html;
return element;
}
function parseHtml(html) {
var template = createTemplate(html);
return createArray(template.content.childNodes);
}
function renderHtml(nodes) {
var html = '';
for (var i = 0; i < nodes.length; ++i) {
var node = nodes[i];
html += node.outerHTML || node.textContent;
}
return html;
}
function iterateChildren(node, callback) {
var nodes = node.childNodes;
for (var i = 0; i < nodes.length; ++i) {
var result = callback(nodes[i]);
if (result === false) {
return false;
}
}
return true;
}
function processElement(node, processText) {
var index = 0;
var result = node.cloneNode(false);
var status = iterateChildren(node, function(child) {
var item = processNode(child, processText);
if (item == null) { // null or undefined
return false;
}
index += 1;
result.appendChild(item);
});
if (status === false && index === 0) {
return null;
}
return result;
}
function processText(node, converter) {
var text = converter(node.nodeValue);
if (text == null) { // null or undefined
return null;
}
return document.createTextNode(text);
}
function processNode(node, converter) {
switch (node.nodeType) {
case Node.ELEMENT_NODE:
return processElement(node, converter);
case Node.TEXT_NODE:
return processText(node, converter);
default:
throw new Error('Unsupported node type.');
}
}
function processNodes(nodes, converter) {
var result = [];
for (var i = 0; i < nodes.length; ++i) {
var item = processNode(nodes[i], converter);
if (item == null) { // null or undefined
break;
}
result.push(item);
}
return result;
}
function isWhiteCharacter(value) {
return value === ' ' || value === '\f' || value === '\n' || value === '\r' || value === '\t' || value === '\v' || value === '\u00a0' || value === '\u1680' || value === '\u2000' || value === '\u2001' || value === '\u2002' || value === '\u2003' || value === '\u2004' || value === '\u2005' || value === '\u2006' || value === '\u2007' || value === '\u2008' || value === '\u2009' || value === '\u200a' || value === '\u2028' || value === '\u2029' || value === '\u202f' || value === '\u205f' || value === '\u3000' || value === '\ufeff';
}
function createTextShortcut(text, limit) {
if (text.length > limit) {
var ending = false;
for (var i = limit - 3; i > -1; --i) {
if (isWhiteCharacter(text[i])) {
ending = true;
} else {
if (ending) {
limit = i + 4;
break;
}
}
}
var part = text.substring(0, limit - 3);
return part + '...';
}
return text;
}
function createNodesShortcut(nodes, limit) {
var count = 0; // stores characters count
var result = processNodes(nodes, function(text) {
if (count < limit) {
count += text.length;
if (count > limit) {
return createTextShortcut(text, text.length - count + limit);
}
return text;
}
return null;
});
return result;
}
function createHtmlShortcut(html, limit) {
var nodes = parseHtml(html);
var result = createNodesShortcut(nodes, limit);
return renderHtml(result);
}
// Usage example:
var inputElement = document.querySelector('#input');
var shortcutElement = document.querySelector('#shortcut');
var shortcutLimit = 50; // limited up to 50 characters
var inputHtml = inputElement.innerHTML.trim();
var shortcutHtml = createHtmlShortcut(inputHtml, shortcutLimit);
shortcutElement.innerHTML = shortcutHtml;
</script>
</body>
</html>