JavaScript - load events order for window, document, dom, body and elements
In this quick article, we are going to discuss about order of load
events.
Kowledge about order helps to execute some logic when DOM is ready, before resources are loaded or after all resources (scripts, styles, images, videos, audio, frames) are loaded. readystatechange
,with readyState="complete"
, body onload
 and window onload
 are designed to work with synchronous loading - it means if some file is loaded asynchronously it will be not signaled in event.
Â
By default load/change events should be executed in following order:
 |
Element (or Object) | Event name | Occurs when: |
1 | Document |
| DOM is parsed but resources are not loaded yet. |
2 | Document | DOMContentLoaded | DOM is parsed but resources are not loaded yet. |
3 | Window | DOMContentLoaded | DOM is parsed but resources are not loaded yet. |
4 | Document |
| DOM is parsed and resources are loaded. |
5 | Image | onload | Image resource is loaded. |
6 | Frame | onload | Frame resource is loaded. |
7 | Body | onload |
DOM is parsed and resources are loaded. (can be handled after window |
8 | Window | onload |
DOM is parsed and resources are loaded. (can be handled before body |
Â
Events comparision example
Note: load events for window and body can be executed in different order - depending of in what order they were added.
// ONLINE-RUNNER:browser;
<!doctype html>
<html>
<head>
<style>
img { width: 200px; height: 200px; }
iframe { border: none; width: 400px; height: 200px; }
</style>
</head>
<body onload="onBodyLoaded()">
<img src="/static/bucket/1574890428058-BZOQxN2D3p--image.png" onload="onImageLoaded()" />
<iframe src="https://dirask.com/about" onload="onFrameLoaded()"></iframe>
<p>Some text ...</p>
<script>
// ------------------------------------------------------------------
// window load events
window.addEventListener('load', function() { // 8 - Window onload
console.log('Window onload event called.');
});
window.addEventListener('DOMContentLoaded', function() { // 3 - Window DOMContentLoaded
console.log('Window DOMContentLoaded event called.');
});
// ------------------------------------------------------------------
// document load events
document.addEventListener('DOMContentLoaded', function() { // 2 - Document DOMContentLoaded
console.log('Document DOMContentLoaded event called.');
});
document.addEventListener('readystatechange', function(e) { // 1 & 4 - Document readystatechange
var state = document.readyState;
console.log('Document readystatechange event called (readyState === "' + state + '").');
});
// ------------------------------------------------------------------
// body load event
function onBodyLoaded() { // 7 - Body onload
console.log('Body onload event called.');
};
// ------------------------------------------------------------------
// image load event
function onImageLoaded() { // 5 - Image onload
console.log('Image onload event called.');
}
// ------------------------------------------------------------------
// iframe load event
function onFrameLoaded() { // 6 - Frame onload
console.log('Frame onload event called.');
}
// ------------------------------------------------------------------
console.log('Script source code (readyState === "' + document.readyState + '").');
</script>
</body>
</html>
Used resources:
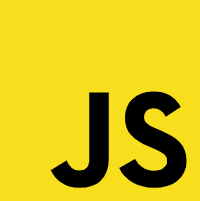
Â