EN
JavaScript - how to make jQuery AJAX POST request with PHP?
2
points
In this article, we would like to show you how to make an AJAX POST request with jQuery to the PHP backend in the following way.
1. jQuery AJAX POST request to PHP backend example
ajax.htm
file:
<!doctype html>
<html lang="en">
<head>
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.4.1/jquery.min.js"></script>
</head>
<body>
<pre id="response"></pre>
<script>
var handle = document.getElementById('response');
$.ajax({
type: 'POST',
url: '/backend.php',
data: {
name: 'John',
age: 25
},
success: function (data) {
handle.innerHTML = 'Response:\n' + data;
},
error: function (jqXHR) {
handle.innerText = 'Error: ' + jqXHR.status;
}
});
</script>
</body>
</html>
backend.php
file:
<?php
if ($_SERVER['REQUEST_METHOD'] === 'POST')
{
echo " Sent data to backend:\n";
echo " + Name: " . $_POST['name'] . "\n";
echo " + Age: " . $_POST['age'] . "\n";
}
else
{
echo " Incorrect request method!";
}
Note:
ajax.htm
andbackend.php
files should be placed on php server both.
Result:
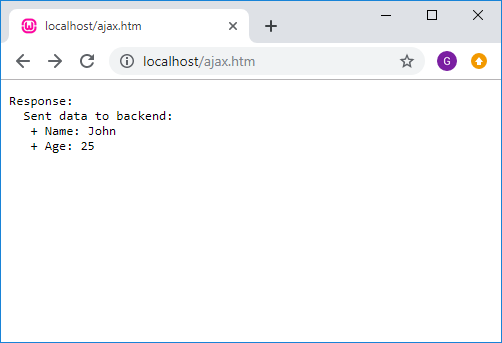
2. jQuery AJAX POST request with alternative API to PHP backend example
ajax.htm
file:
<!doctype html>
<html lang="en">
<head>
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.4.1/jquery.min.js"></script>
</head>
<body>
<pre id="response"></pre>
<script>
var handle = document.getElementById('response');
var data = {
name: 'John',
age: 25
};
$.post('/backend.php', data)
.done(function (data) {
handle.innerHTML = 'Response:\n' + data;
})
.fail(function (jqXHR) {
handle.innerText = 'Error: ' + jqXHR.status;
});
</script>
</body>
</html>
backend.php
file:
<?php
if ($_SERVER['REQUEST_METHOD'] === 'POST')
{
echo " Sent data to backend:\n";
echo " + Name: " . $_POST['name'] . "\n";
echo " + Age: " . $_POST['age'] . "\n";
}
else
{
echo " Incorrect request method!";
}
Note:
ajax.htm
andbackend.php
files should be placed on php server both.
Result:
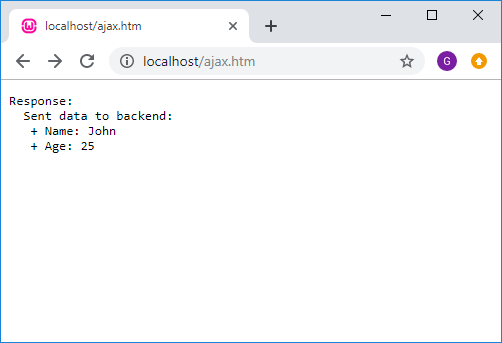