EN
JavaScript - get, set, and remove URL hash parameters
16
points
In this article, we would like to show you how to get, set and remove URL hash parameters in JavaScript using custom logic.
Note: to understand how the below example works, copy the code into your project.
Example index.htm
file:
<!doctype html>
<html>
<head>
<script src="hash-parameters.js"></script>
</head>
<body>
<script>
function doLogic() {
console.log('------- by getParameters() -------');
var parameters = HashParameters.getParameters();
for (var i = 0; i < parameters.length; ++i) {
var parameter = parameters[i];
console.log(parameter.name + ': ' + parameter.value);
}
console.log('------- by getParameter() --------');
var pageNumber = HashParameters.getParameter('page-number', '1');
var pageSize = HashParameters.getParameter('page-size', '20');
var dataOrder = HashParameters.getParameter('data-order', 'A-Z');
console.log('page-number: ' + pageNumber);
console.log('page-size: ' + pageSize);
console.log('data-order: ' + dataOrder);
}
HashParameters.addListener(doLogic); // fired when hash changed
doLogic();
/*
// additional operations
HashParameters.setParameter('page-number', '5');
HashParameters.setParameter('page-size', '50');
HashParameters.removeParameter('page-number');
HashParameters.removeParameter('page-size');
HashParameters.removeParameter('data-order');
HashParameters.clearParameters();
*/
</script>
</body>
</html>
Example result:
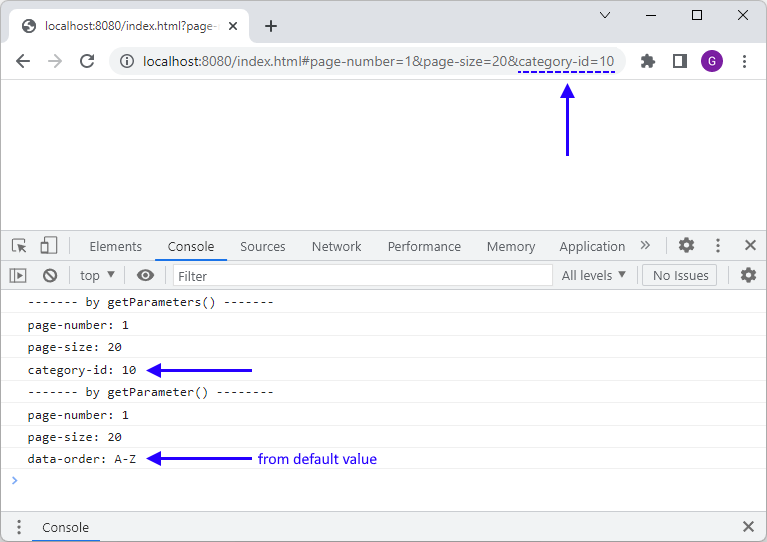
Example hash-parameters.js
file:
// 'use strict';
(function (window) {
var PARAMETER_EXPRESSION = /^([^=]+)(?:=(.*))?$/g;
function removeItem(array, item) {
var index = array.indexOf(item);
if (index == -1) {
return;
}
array.splice(index, 1);
}
function matchParameter(text) {
PARAMETER_EXPRESSION.lastIndex = 0;
return PARAMETER_EXPRESSION.exec(text);
}
function decodeComponent(part) {
if (part) {
return decodeURIComponent(part);
}
return null;
}
function parseParameters(request) {
var parameters = { };
var postfix = request.substring(1);
if (postfix) {
var parts = postfix.split('&');
for (var i = 0; i < parts.length; ++i) {
var part = parts[i];
if (part) {
var match = matchParameter(part);
if (match) {
var name = decodeComponent(match[1]);
parameters[name] = decodeComponent(match[2]);
}
}
}
}
return parameters;
}
function renderHash(parameters) {
var postfix = '';
for (var el in parameters) {
var parameter = parameters[el];
if (postfix) {
postfix += '&';
}
postfix += encodeURIComponent(el);
if (parameter) {
postfix += '=' + encodeURIComponent(parameter);
}
}
return '#' + postfix;
}
window.HashParameters = new function() {
var cache = null; // current hash for changes detection
var parameters = { }; // parsed and decoded parameters
var listeners = [ ]; // hash initialized or typed event methods
function updateParameters() {
var hash = location.hash;
if (hash == cache) {
return false;
}
parameters = parseParameters(cache = hash);
return true;
}
function updateHash() {
var hash = renderHash(parameters);
if (hash == cache) {
return false;
}
location.hash = cache = hash;
return true;
}
function fireListeners() {
for (var i = 0; i < listeners.length; ++i) {
var listener = listeners[i];
listener.call(window);
}
}
window.addEventListener('hashchange', function () {
if (updateParameters()) {
fireListeners();
}
});
updateParameters();
// public methods
this.addListener = function(action) {
if (action instanceof Function) {
listeners.push(action);
var result = function() {
if (action) {
removeItem(listeners, action);
action = null;
}
};
return result;
}
throw new Error('Indicated listener action is not function type.');
};
this.removeListener = function(action) {
removeItem(listeners, action);
};
this.getParameters = function() {
var result = [ ];
for (var key in parameters) {
result.push({name: key, value: parameters[key]});
}
return result;
};
this.getParameter = function(name, defaultValue) {
return parameters[name] || defaultValue;
};
this.setParameter = function(name, value) {
parameters[name] = value;
updateHash();
};
this.removeParameter = function(name) {
delete parameters[name];
updateHash();
};
this.clearParameters = function() {
parameters = { };
updateHash();
};
};
})(window);