EN
JavaScript - get element by id
2
points
In this article, we would like to show you how to get an element handle by id in JavaScript.
1. document.getElementById
method example
First example
// ONLINE-RUNNER:browser;
<!doctype html>
<html>
<body>
<div id="my-element">Some text here...</div>
<script>
var handle = document.getElementById('my-element');
handle.style.background = 'red';
</script>
</body>
</html>
Result:
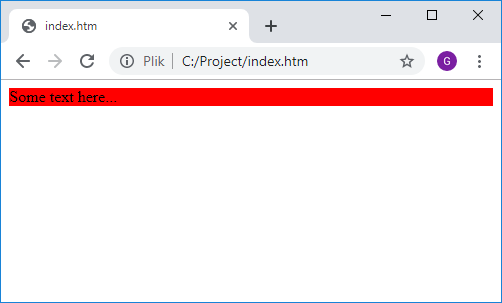
Second example
// ONLINE-RUNNER:browser;
<!doctype html>
<html>
<body>
<div id="exampleId">Original text.</div>
<button onclick="changeText()">Change Text Button</button>
<script>
var element = document.getElementById('exampleId');
function changeText() {
element.innerHTML = "Changed text.";
}
</script>
</body>
</html>
2. document.querySelector
method example
// ONLINE-RUNNER:browser;
<!doctype html>
<html>
<body>
<div id="my-element">Some text here...</div>
<script>
var handle = document.querySelector('#my-element');
handle.style.background = 'red';
</script>
</body>
</html>
Result:
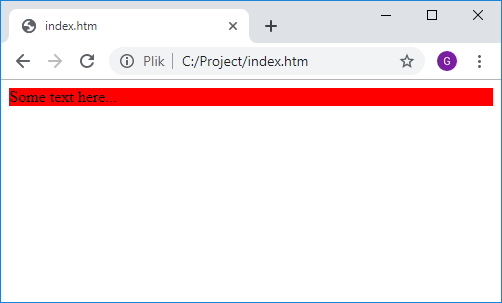
Note:
document.querySelector
method uses css selectors - it means each id name should be prefixed with hash.
3. document.querySelectorAll
method example
Writing web page it is possible to define few elements with same id attribute - this aproach is strongly not recommended because of
id
was designed to be unique.
// ONLINE-RUNNER:browser;
<!doctype html>
<html>
<body>
<div id="my-element">Some text here...</div>
<div id="my-element">Some text here...</div>
<div id="my-element">Some text here...</div>
<script>
var handles = document.querySelectorAll('#my-element');
for(var i = 0; i < handles.length; ++i)
handles[i].style.background = 'red';
</script>
</body>
</html>
Result:
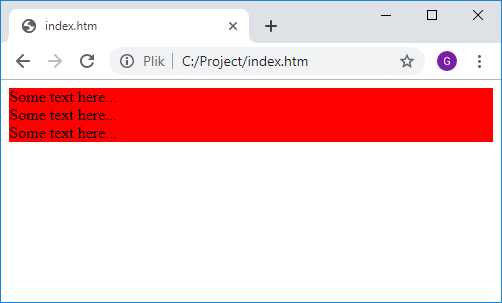