EN
JavaScript - draw point on canvas element
10
points
In this article, we would like to show how to draw points on canvas element using JavaScript.
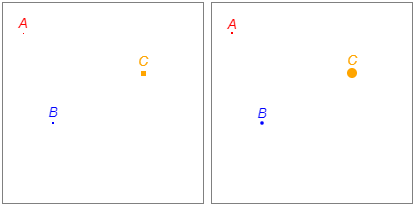
1. Squared points
To draw squared points we can use fillRect()
method.
// ONLINE-RUNNER:browser;
<!doctype html>
<html>
<head>
<style>
#my-canvas { border: 1px solid gray; }
</style>
</head>
<body>
<canvas id="my-canvas" width="200" height="200"></canvas>
<script>
function drawPoint(context, x, y, label, color, size) {
if (color == null) {
color = '#000';
}
if (size == null) {
size = 5;
}
var radius = 0.5 * size;
// to increase smoothing for numbers with decimal part
var pointX = Math.round(x - radius);
var pointY = Math.round(y - radius);
context.beginPath();
context.fillStyle = color;
context.fillRect(pointX, pointY, size, size);
context.fill();
if (label) {
var textX = Math.round(x);
var textY = Math.round(pointY - 5);
context.font = 'Italic 14px Arial';
context.fillStyle = color;
context.textAlign = 'center';
context.fillText(label, textX, textY);
}
}
// Usage example:
var canvas = document.querySelector('#my-canvas');
var context = canvas.getContext('2d');
drawPoint(context, 20, 30, 'A', 'red', 1);
drawPoint(context, 50, 120, 'B', 'blue', 2);
drawPoint(context, 140, 70, 'C', 'orange', 5);
</script>
</body>
</html>
2. Rounded points
To draw rounded points we can use arc()
method.
// ONLINE-RUNNER:browser;
<!doctype html>
<html>
<head>
<style>
#my-canvas { border: 1px solid gray; }
</style>
</head>
<body>
<canvas id="my-canvas" width="200" height="200"></canvas>
<script>
function drawPoint(context, x, y, label, color, size) {
if (color == null) {
color = '#000';
}
if (size == null) {
size = 5;
}
// to increase smoothing for numbers with decimal part
var pointX = Math.round(x);
var pointY = Math.round(y);
context.beginPath();
context.fillStyle = color;
context.arc(pointX, pointY, size, 0 * Math.PI, 2 * Math.PI);
context.fill();
if (label) {
var textX = pointX;
var textY = Math.round(pointY - size - 3);
context.font = 'Italic 14px Arial';
context.fillStyle = color;
context.textAlign = 'center';
context.fillText(label, textX, textY);
}
}
// Usage example:
var canvas = document.querySelector('#my-canvas');
var context = canvas.getContext('2d');
drawPoint(context, 20, 30, 'A', 'red', 1);
drawPoint(context, 50, 120, 'B', 'blue', 1.7);
drawPoint(context, 140, 70, 'C', 'orange', 5);
</script>
</body>
</html>