EN
JavaScript - draw line on canvas element?
8
points
In this short article, we would like to show how to draw line on canvas element using JavaScript.
Simple solution:
// line from x1=10, y1=50 to x2=170, y2=150
context.beginPath();
context.moveTo(10, 50);
context.lineTo(170, 150);
context.stroke();
Preview:
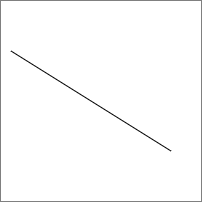
Practical example
Using JavaScript it is possible to draw line in the following way:
// ONLINE-RUNNER:browser;
<!doctype html>
<html>
<head>
<style>
#my-canvas { border: 1px solid gray; }
</style>
</head>
<body>
<canvas id="my-canvas" width="200" height="200"></canvas>
<script>
function drawLine(context, x1, y1, x2, y2) {
context.beginPath();
context.moveTo(x1, y1);
context.lineTo(x2, y2);
context.stroke();
}
// Usage example:
var canvas = document.querySelector('#my-canvas');
var context = canvas.getContext('2d');
drawLine(context, 10, 50, 170, 150); // context, x1, y1, x2, y2
</script>
</body>
</html>