EN
Java - find differences on two images (absolute difference)
5 points
In this short article, we would like to show how to find differences on two images using Java.
To find differences on two images it is good to use absolute subtraction on two images. Let's suppose we have 2 images: A
and B
. The result image (C
image) may be calculated using fomula: |B - A| = C
.
Operation visualization:
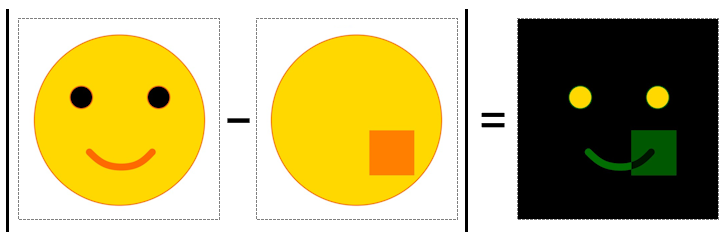
Result interpretation:
Black colors mean: pixels do not have difference. White colors mean: pixels are totally different. Middle colors mean: there is some difference (smaller in direction to black, bigger in direction to white).
Note: to reverse colors (from white to black) we can use fomula:
255 - |B - A| = C
what was presented in this article.
Practical example:
xxxxxxxxxx
1
package com.example;
2
3
import java.awt.Color;
4
import java.awt.image.BufferedImage;
5
import java.io.File;
6
import java.io.IOException;
7
8
import javax.imageio.ImageIO;
9
10
public class Program {
11
12
public static void main(String[] args) throws IOException {
13
BufferedImage aImage = ImageIO.read(new File("a.png")); // A image (input image)
14
BufferedImage bImage = ImageIO.read(new File("b.png")); // B image (input image)
15
int aWidth = aImage.getWidth();
16
int aHeight = aImage.getHeight();
17
if (aWidth != bImage.getWidth() || aHeight != bImage.getHeight()) {
18
throw new IOException("Input images doesn't have the same size.");
19
}
20
BufferedImage cImage = new BufferedImage(aWidth, aHeight, BufferedImage.TYPE_INT_RGB);
21
for (int y = 0; y < aHeight; ++y) {
22
for (int x = 0; x < aWidth; ++x) {
23
Color aColor = new Color(aImage.getRGB(x, y));
24
Color bColor = new Color(bImage.getRGB(x, y));
25
Color cColor = new Color(Math.abs(bColor.getRed() - aColor.getRed()), Math.abs(bColor.getGreen() - aColor.getGreen()), Math.abs(bColor.getBlue() - aColor.getBlue()));
26
cImage.setRGB(x, y, cColor.getRGB());
27
}
28
}
29
ImageIO.write(cImage, "PNG", new File("c.png")); // C image (output image)
30
}
31
}
a.png
file:
b.png
file: