EN
HTML - add css to html
19 points
In this article, we would like to show how to add styles to HTML code (how to add CSS to HTML).
The most popular approaches are: using link
tag, style
tag and style
attribute.
Quick solutions:
1. Link to stylesheet file (*.css
file):
xxxxxxxxxx
1
<link rel="stylesheet" type="text/css" href="/path/to/styles.css" />
Hint: put it to
<head>
element.
2. Embedded styles:
xxxxxxxxxx
1
<style>
2
3
body {
4
background: red;
5
}
6
7
</style>
Hint: put it somewhere in the source code.
3. Inline styles:
xxxxxxxxxx
1
<p style="font-size: 20px; color: brown;">Brown text ...</p>
This article is like an overview of different approaches with examples.
index.htm
file:
xxxxxxxxxx
1
2
<html>
3
<head>
4
<link rel="stylesheet" type="text/css" href="styles.css" />
5
</head>
6
<body>
7
Red web page...
8
</body>
9
</html>
styles.css
file:
xxxxxxxxxx
1
body {
2
background: red;
3
}
Result:
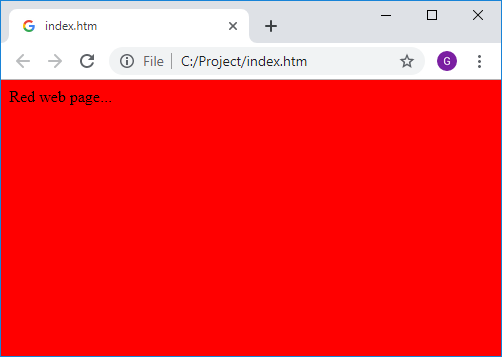
index.htm
file:
xxxxxxxxxx
1
2
<html>
3
<head>
4
<style>
5
6
body {
7
background: red;
8
}
9
10
</style>
11
</head>
12
<body>
13
Red web page...
14
</body>
15
</html>
Result:
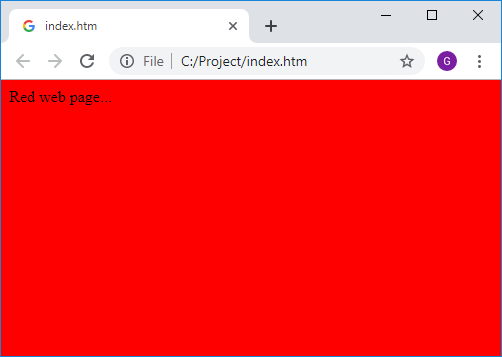
index.htm
file:
xxxxxxxxxx
1
2
<html>
3
<body style="background: red;">
4
<p style="font-size: 20px; color: brown;">
5
Red web page...
6
</p>
7
</body>
8
</html>
Result:
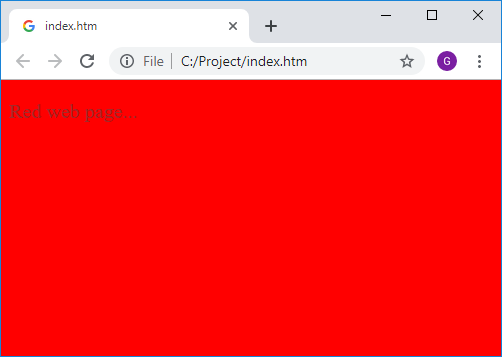
index.htm
file:
xxxxxxxxxx
1
2
<html>
3
<head>
4
<style>
5
6
@import 'styles.css';
7
8
</style>
9
</head>
10
<body>
11
This is red web page...
12
</body>
13
</html>
styles.css
file:
xxxxxxxxxx
1
body {
2
background: red;
3
}
Result:
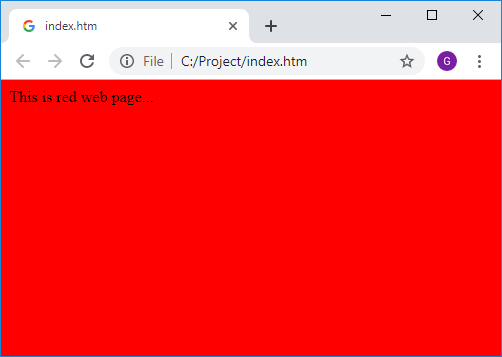
index.htm
file:
xxxxxxxxxx
1
2
<html>
3
<body>
4
<p>This is red web page...</p>
5
<script>
6
7
var element = document.createElement('link');
8
9
element.addEventListener('load', function() {
10
document.body.innerHTML += '<p>Styles loaded!</p>';
11
});
12
13
element.addEventListener('error', function() {
14
document.body.innerHTML += '<p>Styles error!</p>';
15
});
16
17
element.setAttribute('rel', 'stylesheet');
18
element.setAttribute('href', 'styles.css');
19
20
document.head.appendChild(element);
21
22
</script>
23
</body>
24
</html>
styles.css
file:
xxxxxxxxxx
1
body {
2
background: red;
3
}
Result:
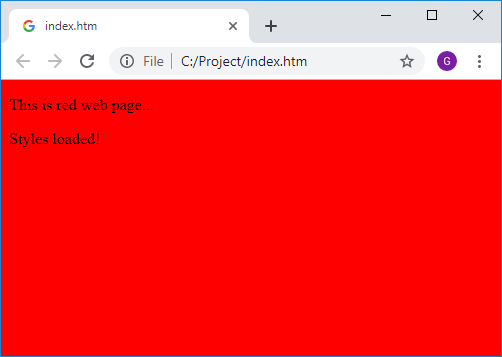
index.htm
file:
xxxxxxxxxx
1
2
<html>
3
<body>
4
<p>This is red web page...</p>
5
<script>
6
7
var element = document.createElement('style');
8
9
element.appendChild(document.createTextNode('body { background: red; }'));
10
// Add more rules here ...
11
12
document.head.appendChild(element);
13
14
</script>
15
</body>
16
</html>
Result:
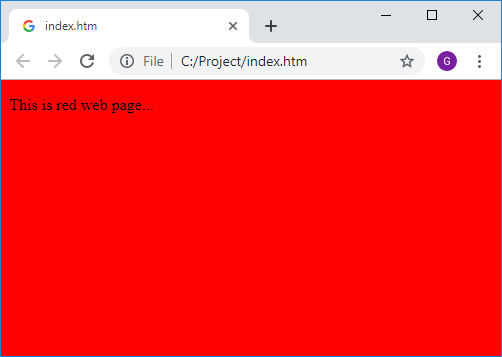
index.htm
file:
xxxxxxxxxx
1
2
<html>
3
<body id="page">
4
<p>This is red web page...</p>
5
<script>
6
7
var element = document.getElementById('page'); // or document.body
8
9
element.style.background = 'red';
10
element.style.color = 'brown';
11
//element.style.cssText = 'background: red; color: brown;';
12
13
</script>
14
</body>
15
</html>
Result:
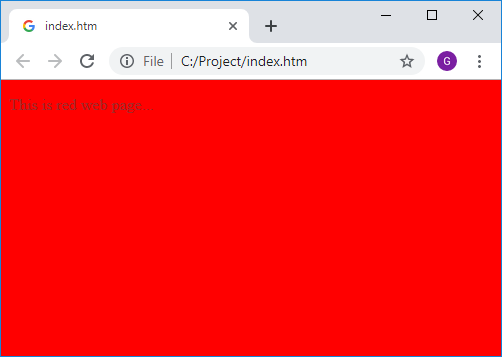
Alternative titles
- HTML - how to attach css to html?
- HTML - how to add css file to html?
- HTML - how to add style file to html?
- HTML - how to attach style file to html?
- HTML - different ways how to attach style to html?
- HTML - different ways how to add style file to html?
- HTML - how to include css to html?
- HTML - how to import css to html?
- HTML - how to import style to html?