EN
JavaScript - script loading error event
0 points
In this article, we would like to show you the script loading error event in JavaScript.
Quick solution:
xxxxxxxxxx
1
<script>
2
var script = document.createElement('script');
3
script.onerror = function () {
4
console.log('script loading error.');
5
};
6
script.src = '/incorrect/path/to/script.js';
7
document.head.append(script);
8
</script>
In this example, we create index.html
with incorrect src
property and add the onerror
event to display a message on script loading error.
xxxxxxxxxx
1
2
<html>
3
<body>
4
<script>
5
var script = document.createElement('script');
6
script.onerror = function() {
7
alert('script loading error.');
8
};
9
script.src = '/incorrect/path/to/script.js';
10
document.head.appendChild(script);
11
</script>
12
</body>
13
</html>
Result:
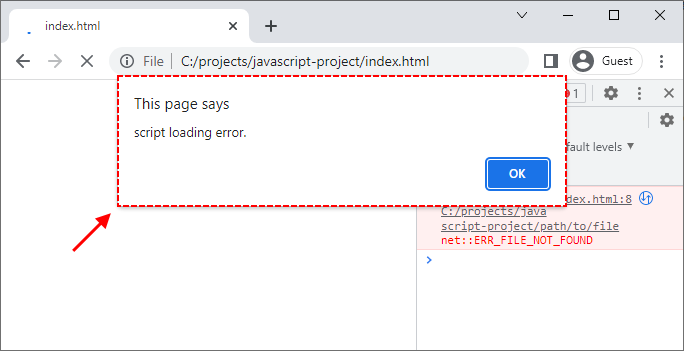
In this example, we add a function to the script element onerror
property to handle the script loading error.
xxxxxxxxxx
1
2
<html>
3
<head>
4
<script>
5
function handleError() {
6
console.log('Script loading error.');
7
}
8
</script>
9
<script src="/incorrect/path/to/script.js" onerror="handleError()"></script>
10
</head>
11
<body> Web page body... </body>
12
</html>