EN
JavaScript - convert textarea lines into array
0 points
In this article, we would like to show you how to convert textarea lines into an array using JavaScript.
Quick solution:
xxxxxxxxxx
1
// get the value of textarea by id:
2
var textareaValue = document.querySelector('#textareaId').value;
3
4
// split value of textarea by \n (it will be converted into array):
5
var linesArray = textareaValue.split('\n');
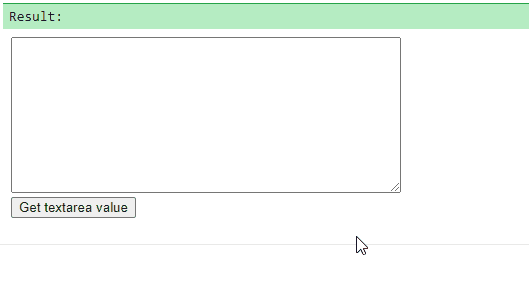
In this example, we get handle to textarea
by id using document.querySelector()
method. Then we take its value
and we split it into an array by new line character (\n
) using split()
method.
xxxxxxxxxx
1
2
<html>
3
<head>
4
<style>
5
// ...
6
</style>
7
</head>
8
<body>
9
<textarea id='myTextArea'
10
rows='10'
11
cols='50'></textarea>
12
<br>
13
<button onclick="handleClick()">Get textarea value</button>
14
<script>
15
16
function handleClick() {
17
// get the value of textarea:
18
var textareaValue = document.querySelector('#myTextArea').value;
19
20
// split value of textarea by \n (it will be converted into array):
21
var linesArray = textareaValue.split('\n');
22
console.log(JSON.stringify(linesArray));
23
}
24
25
</script>
26
</body>
27
</html>