EN
JavaScript - resize JPG image (Vanilla JS)
4 points
In this article, we would like to show how to resize or reduce image size in pure JavaScript using canvas.
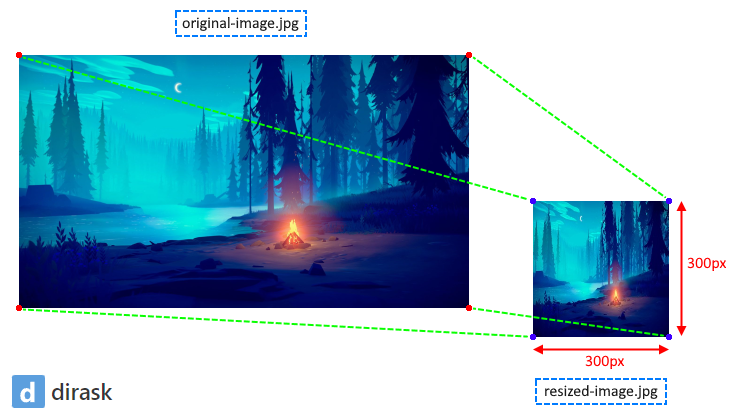
Practical example:
xxxxxxxxxx
1
2
<html>
3
<body>
4
<img id="dst-image">
5
<script>
6
7
function resizeImage$1(imageElement, newWidth, newHeight, newQuality) {
8
var canvas = document.createElement('canvas');
9
canvas.width = newWidth;
10
canvas.height = newHeight;
11
var context = canvas.getContext('2d');
12
context.drawImage(imageElement, 0, 0, newWidth, newHeight);
13
try {
14
return canvas.toDataURL('image/jpeg', newQuality);
15
} catch (e) {
16
return null;
17
}
18
};
19
20
function resizeImage$2(imageUrl, newWidth, newHeight, newQuality, onReady, onError) {
21
var image = document.createElement('img');
22
image.onload = function() {
23
var dataUrl = resizeImage$1(image, newWidth, newHeight, newQuality);
24
if (dataUrl) {
25
onReady(dataUrl);
26
} else {
27
if (onError) {
28
onError('Image saving error.');
29
}
30
}
31
};
32
image.onerror = function() {
33
if (onError) {
34
onError('Image loading error.');
35
}
36
};
37
image.src = imageUrl;
38
};
39
40
41
// Usage example:
42
43
var srcUrl = 'https://dirask.com/static/bucket/1655940866050-LbA292PD9v--original-image.jpg';
44
45
var newWidth = 300;
46
var newHeight = 300;
47
var newQuality = 0.9; // should be from 0 to 1.0
48
49
function onReady(dataUrl) {
50
var dstImage = document.querySelector('#dst-image');
51
dstImage.src = dataUrl;
52
}
53
function onError(message) {
54
console.error(message);
55
}
56
57
resizeImage$2(srcUrl, newWidth, newHeight, newQuality, onReady, onError);
58
59
</script>
60
</body>
61
</html>
Used resource: