EN
C# / .NET - create directory
3 points
In this article, we would like to show you how to create directory in C#.
Quick solution:
xxxxxxxxxx
1
string path = @"C:\path\to\directory";
2
3
if (!Directory.Exists(path))
4
Directory.CreateDirectory(path);
Warning: the above source code may thrown some exceptions, so it is good to catch or forward them.
In this example, we use Directory.CreateDirectory()
method to create directory if it doesn't exist yet.
xxxxxxxxxx
1
using System;
2
using System.IO;
3
4
public static class Program
5
{
6
public static void Main(string[] args)
7
{
8
string path = @"C:\Users\Username\Desktop\example\dir1";
9
10
try
11
{
12
if (Directory.Exists(path)) // checks if the directory exists
13
Console.WriteLine("That directory already exists.");
14
15
else
16
{
17
DirectoryInfo directory= Directory.CreateDirectory(path); // creates a directory
18
19
Console.WriteLine("The directory was created successfully.");
20
}
21
}
22
catch (Exception e)
23
{
24
Console.WriteLine("The operation failed: {0}", e.ToString());
25
}
26
}
27
}
Result:
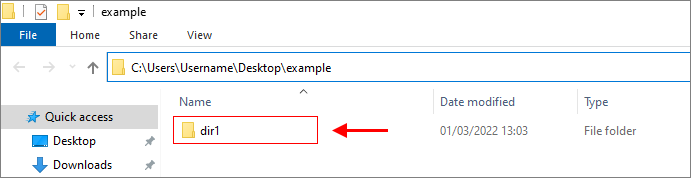