EN
PHP - receive json POST request
9
points
In this article, we would like to show you how to receive json requests in PHP.
Parse JSON input stream example
backend.php
file:
<?php
function parseInput()
{
$data = file_get_contents("php://input");
if($data == false)
return null;
return json_decode($data);
}
if($_SERVER['REQUEST_METHOD'] === 'POST')
{
$data = parseInput();
echo " POST request method\n";
echo " + Name: " . $data->name . "\n";
echo " + Age: " . $data->age . "\n";
}
else
{
echo "Unsupported request method.";
}
Note: to use different request method read this article.
ajax.htm
file:
<!doctype html>
<html lang="en">
<head>
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.4.1/jquery.min.js"></script>
</head>
<body>
<pre id="response"></pre>
<script>
var handle = document.getElementById('response');
var data = {
name: 'John',
age: 25
};
$.ajax({
type: 'POST',
url: '/backend.php',
data: JSON.stringify(data),
success: function (data) {
handle.innerHTML = 'Response:\n' + data;
},
error: function(error) {
handle.innerText = 'Error: ' + error.status;
}
});
</script>
</body>
</html>
Result:
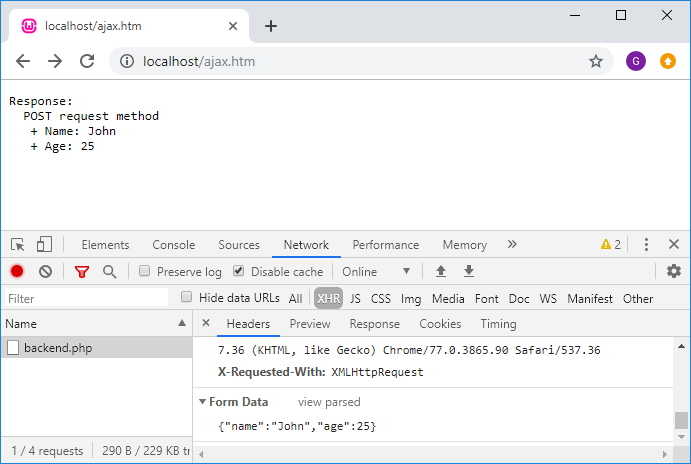