EN
Node.js - PostgreSQL Find rows between two timestamps
0
points
In this article, we would like to show you how to find rows between two absoluteΒ timestamps in the Postgres database from Node.js level.
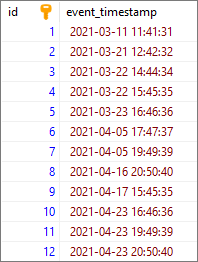
Note:Β at the end of this article you can find database preparation SQL queries.
const pg = require('pg');
const types = pg.types;
types.setTypeParser(1114, (stringValue) => {
return stringValue;
});
const client = new pg.Client({
host: '127.0.0.1',
user: 'my_username',
database: 'my_database',
password: 'my_password',
port: 5432,
});
const fetchEventsBetween = async (fromDate, toDate) => {
const query = `
SELECT *
FROM "events"
WHERE "event_timestamp" BETWEEN $1 AND $2
`;
await client.connect(); // creates connection
try {
const { rows } = await client.query(query, [fromDate, toDate]); // sends query
return rows;
} finally {
await client.end(); // closes connection
}
};
fetchEventsBetween('2021-03-22', '2021-04-17')
.then(result => console.table(result))
.catch(error => console.error(error.stack));
Result:
βββββββββββ¬βββββ¬ββββββββββββββββββββββββ
β (index) β id β event_timestamp β
βββββββββββΌβββββΌββββββββββββββββββββββββ€
β 0 β 3 β '2021-03-22 14:44:34' β
β 1 β 4 β '2021-03-22 15:45:35' β
β 2 β 5 β '2021-03-23 16:46:36' β
β 3 β 6 β '2021-04-05 17:47:37' β
β 4 β 7 β '2021-04-05 19:49:39' β
β 5 β 8 β '2021-04-16 20:50:40' β
βββββββββββ΄βββββ΄ββββββββββββββββββββββββ
Database preparation
create_tables.sql
Β file:
CREATE TABLE "events" (
"id" SERIAL PRIMARY KEY,
"event_timestamp" TIMESTAMP NOT NULL
);
insert_data.sql
Β file:
INSERT INTO "events"
("event_timestamp")
VALUES
('2021-03-11 11:41:31'),
('2021-03-21 12:42:32'),
('2021-03-22 14:44:34'),
('2021-03-22 15:45:35'),
('2021-03-23 16:46:36'),
('2021-04-05 17:47:37'),
('2021-04-05 19:49:39'),
('2021-04-16 20:50:40'),
('2021-04-17 15:45:35'),
('2021-04-23 16:46:36'),
('2021-04-23 19:49:39'),
('2021-04-23 20:50:40');
Native SQL query (used in the above example):
SELECT *
FROM "events"
WHERE "event_timestamp" BETWEEN '2021-03-22' AND '2021-04-17'