EN
JavaScript - Rectilinear Distance function
10 points
In this short article, we would like to show how to calculate Rectilinear Distance using JavaScript.
The universal distance formula is: Where:
|
The two-dimensional formula is: The three-dimensional formula is: |
Rectilinear Distance is also known as taxicab metric, L1 distance or L1 norm, snake distance, city block distance, Manhattan distance, or Manhattan length.
It was used to measure the absolute distance needed to move from one place to the second one in taxicab city.
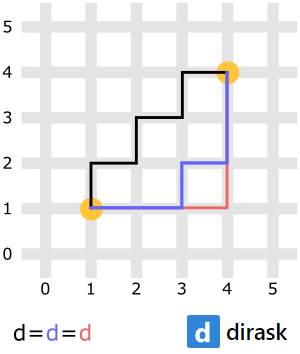
A practical example in 2D:
xxxxxxxxxx
1
const calculateRectilinearDistance = (a, b) => {
2
if (a.length === 0 || a.length !== b.length) {
3
return NaN;
4
}
5
let sum = 0.0;
6
for (let i = 0; i < a.length; ++i) {
7
sum += Math.abs(a[i] - b[i]);
8
}
9
return sum;
10
};
11
12
13
// Usage example:
14
15
const a = [1, 1];
16
const b = [4, 4];
17
18
const distance = calculateRectilinearDistance(a, b);
19
20
console.log(distance); // 6
In this section, you can find Rectilinear Distance examples for 3D and 4D.
xxxxxxxxxx
1
const calculateRectilinearDistance = (a, b) => {
2
if (a.length === 0 || a.length !== b.length) {
3
return NaN;
4
}
5
let sum = 0.0;
6
for (let i = 0; i < a.length; ++i) {
7
sum += Math.abs(a[i] - b[i]);
8
}
9
return sum;
10
};
11
12
13
// Usage example:
14
15
16
const a1 = [1, 2, 3];
17
const b1 = [5, 5, 5];
18
19
const distance1 = calculateRectilinearDistance(a1, b1);
20
21
console.log(distance1); // 9
22
23
24
const a2 = [1, 2, 3, 4];
25
const b2 = [3, 4, 4, 3];
26
27
const distance2 = calculateRectilinearDistance(a2, b2);
28
29
console.log(distance2); // 6