EN
Next.js - get dynamic route segment value
0 points
In this article, we would like to show you how to get dynamic route segments in Next.js.
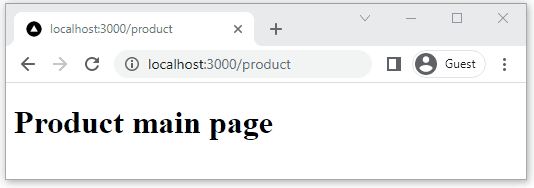
In order to access dynamic route segments, we need to use the useRouter()
hook provided by the next/router
module. With the useRouter()
hook we can access router.query
that contains parsed query string parameters.
Below, you can see the example of how to get a dynamic segment with the name id
by destructuring it from the query
object:
xxxxxxxxxx
1
import { useRouter } from 'next/router';
2
3
function Product() {
4
const router = useRouter();
5
const { id } = router.query; // gets id route segment specified in [id].js filename
6
7
return <h1>Product {id} details</h1>;
8
}
9
10
export default Product;
Note:
The dynamic segments are passed as strings.
xxxxxxxxxx
1
/next.js-project/
2
├─ next/
3
├─ node_modules/
4
└─ pages/
5
├─ api/
6
└─ product/
7
├─ index.js
8
└─ [id].js
product/index.js
:
xxxxxxxxxx
1
function Product() {
2
return <h1>Product main page</h1>;
3
}
4
5
export default Product;
product/[id].js
:
xxxxxxxxxx
1
import { useRouter } from 'next/router';
2
3
function Product() {
4
const router = useRouter();
5
const { id } = router.query; // gets id route segment specified in [id].js filename
6
7
return <h1>Product: {id}</h1>;
8
}
9
10
export default Product;