EN
Youtube - print all video ids in browser console using JavaScript in channel video view (without saved time query parameter)
4
points
In this article we will see how to get youtube video id using JavaScript and browser console.
Quick solution:
[...document.querySelectorAll('a#video-title')]
.map(entry => {
const expression = /[?&]v=([^&]+)/i;
const matching = expression.exec(entry.href);
if (matching) {
return decodeURIComponent(matching[1]);
}
return link;
});
Or:
[...document.querySelectorAll('#video-title')]
.map(entry => {
let url = new URL(entry.href);
return url.searchParams.get('v');
});
Or:
[...document.querySelectorAll('#video-title')]
.map(entry => {
let link = entry.href.replace('https://www.youtube.com/watch?v=', '');
let timeIndex = link.indexOf('&');
if (timeIndex != -1) {
return link.substring(0, timeIndex);
}
return link;
});
// Sample output:
// 0: "lBPOEZLMdd4"
// 1: "2OoQMLXGiyw"
// 2: "l4qB24OSjx8"
// ...
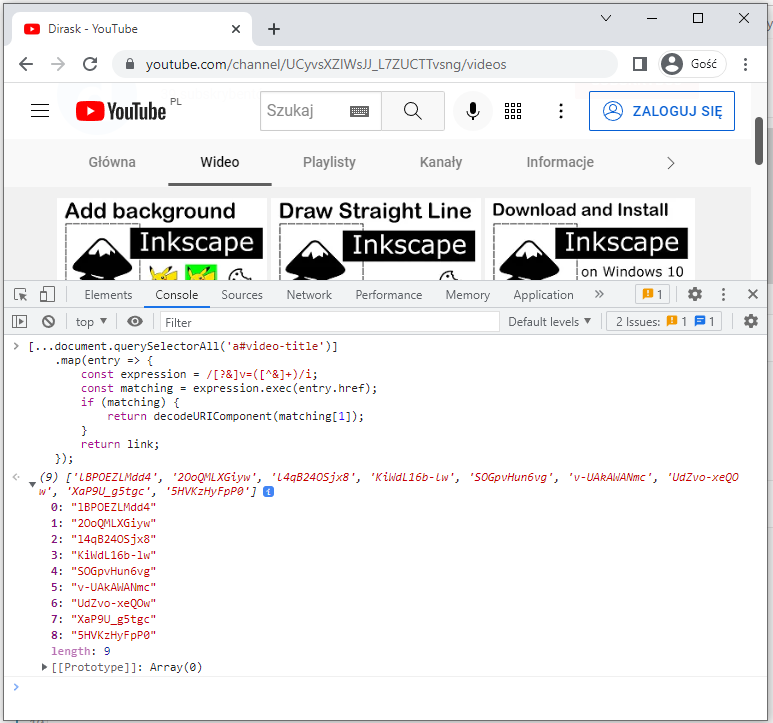
Youtube video url analysis:
// youtube video url with video ID and
https://www.youtube.com/watch?v=lBPOEZLMdd4
// Video ID: lBPOEZLMdd4
// youtube video url with video ID and time query parameter set to 22 seconds:
https://www.youtube.com/watch?v=lBPOEZLMdd4&t=22s
// Video ID: lBPOEZLMdd4
// Time where we stopped watching: 22s
Steps
Below in couple of steps we will see how to get video ids.
Step 1
Go to youtube channel and enter videos, for example Dirask youtube channel - videos:
Step 2
Open browser console (for this tutorial we've used Chrome Browser and Chrome DevTools)
Shortcuts:
- Press F12 - it will open DevTools
- Press Escape - it will open console
Step 3 - Print all links with time query parameter:
[...document.querySelectorAll('#video-title')].map(entry => entry.href);
Output:
0: "https://www.youtube.com/watch?v=lBPOEZLMdd4&t=2s"
1: "https://www.youtube.com/watch?v=2OoQMLXGiyw"
2: "https://www.youtube.com/watch?v=l4qB24OSjx8&t=1s"
3: "https://www.youtube.com/watch?v=KiWdL16b-lw&t=25s"
4: "https://www.youtube.com/watch?v=SOGpvHun6vg"
5: "https://www.youtube.com/watch?v=v-UAkAWANmc"
6: "https://www.youtube.com/watch?v=UdZvo-xeQOw"
7: "https://www.youtube.com/watch?v=XaP9U_g5tgc"
8: "https://www.youtube.com/watch?v=5HVKzHyFpP0"
Step 4 - Print all video ids (with time query parameter)
[...document.querySelectorAll('#video-title')]
.map(entry => {
let link = entry.href.replace('https://www.youtube.com/watch?v=', '');
return link;
});
Output:
0: "lBPOEZLMdd4&t=2s"
1: "2OoQMLXGiyw"
2: "l4qB24OSjx8&t=1s"
3: "KiWdL16b-lw&t=25s"
4: "SOGpvHun6vg"
5: "v-UAkAWANmc"
6: "UdZvo-xeQOw"
7: "XaP9U_g5tgc"
8: "5HVKzHyFpP0"
Step 5 - Print all video ids (without time query parameter)
[...document.querySelectorAll('#video-title')]
.map(entry => {
let link = entry.href.replace('https://www.youtube.com/watch?v=', '');
let timeIndex = link.indexOf('&');
if (timeIndex != -1) {
return link.substring(0, timeIndex);
}
return link;
});
Output:
0: "lBPOEZLMdd4"
1: "2OoQMLXGiyw"
2: "l4qB24OSjx8"
3: "KiWdL16b-lw"
4: "SOGpvHun6vg"
5: "v-UAkAWANmc"
6: "UdZvo-xeQOw"
7: "XaP9U_g5tgc"
8: "5HVKzHyFpP0"