EN
React - use PayPal donate button
10 points
In this short article, we would like to show how to use PayPal donate button in React.
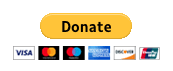
Hint: to use PayPal donate button you need to have business account (business account creation is for free)
It is necessary to do few steps:
- Register donate button in PayPal panel (check this link),
- Integrate below source code with your site:
- add
https://www.paypalobjects.com/donate/sdk/donate-sdk.js
script at the end of your tempalte, - update
DonateButton
useEffect
source code using generated source code in PayPal panel or just copyhosted_button_id: 'PUT_YOUR_BUTTON_ID_HERE'
.
- add
template.html
file:
xxxxxxxxxx
1
2
<html>
3
<head>
4
<!-- Site head elements here... -->
5
</head>
6
<body>
7
<!-- React root element here ... -->
8
<script src="https://www.paypalobjects.com/donate/sdk/donate-sdk.js" charset="UTF-8"></script>
9
</body>
10
</html>
DonateButton.jsx
file:
xxxxxxxxxx
1
import React, { useEffect, useMemo } from 'react';
2
3
let counter = 0;
4
5
const generateId = () => {
6
return `ID-${++counter}`; // if it is necessary, use some better unique id generator
7
};
8
9
const DonateButton = () => {
10
const buttonRef = useRef(null);
11
const buttonId = useMemo(() => `ID-${generateId()}`, []);
12
useEffect(() => {
13
const button = window.PayPal.Donation.Button({
14
env: 'production',
15
hosted_button_id: 'PUT_YOUR_BUTTON_ID_HERE',
16
image: {
17
src: 'https://www.paypalobjects.com/en_US/i/btn/btn_donateCC_LG.gif',
18
alt: 'Donate with PayPal button',
19
title: 'PayPal - The safer, easier way to pay online!',
20
}
21
});
22
button.render(`#${buttonRef.current.id}`); // you can change the code and run it when DOM is ready
23
}, []);
24
return (
25
<div ref={buttonRef} id={buttonId} />
26
);
27
};
28
29
export default DonateButton;
Hint: change
PUT_YOUR_BUTTON_ID_HERE
value to id available in your PayPal panel.
Usage example:
xxxxxxxxxx
1
import React from 'react';
2
3
import DonateButton from './DonateButton';
4
5
const App = () => {
6
return (
7
<div>
8
<DonateButton />
9
</div>
10
);
11
};
12
13
const root = document.querySelector('#root');
14
ReactDOM.render(<App />, root);