EN
JavaScript - get method call stack
4 points
In this short article we want to show how to get method call stack in JavaScript in Google Chrome web browser.
Quick solution:
xxxxxxxxxx
1
const pattern = /^\s*at\s*(\w*)\s+\(?(.*)\)?\s*$/g;
2
3
const getCallStack = () => {
4
const stack = [];
5
try {
6
throw new Error(); // exception throwing to get access to call stack
7
} catch(e) {
8
const parts = e.stack.split('\n');
9
for (let i = 2; i < parts.length; ++i) {
10
pattern.lastIndex = 0;
11
const match = pattern.exec(parts[i]);
12
if (match) {
13
stack.push({
14
method: match[1],
15
file: match[2]
16
});
17
}
18
}
19
}
20
return stack;
21
};
22
23
// Usage example:
24
25
const method1 = () => {
26
const stack = getCallStack();
27
console.log('----------------------------');
28
console.log(' method1 ');
29
console.log('----------------------------');
30
console.log(JSON.stringify(stack[0], null, 2));
31
console.log(JSON.stringify(stack[1], null, 2));
32
console.log();
33
};
34
35
const method2 = () => {
36
const stack = getCallStack();
37
console.log('----------------------------');
38
console.log(' method2 ');
39
console.log('----------------------------');
40
console.log(JSON.stringify(stack[0], null, 2));
41
console.log();
42
method1();
43
};
44
45
method2();
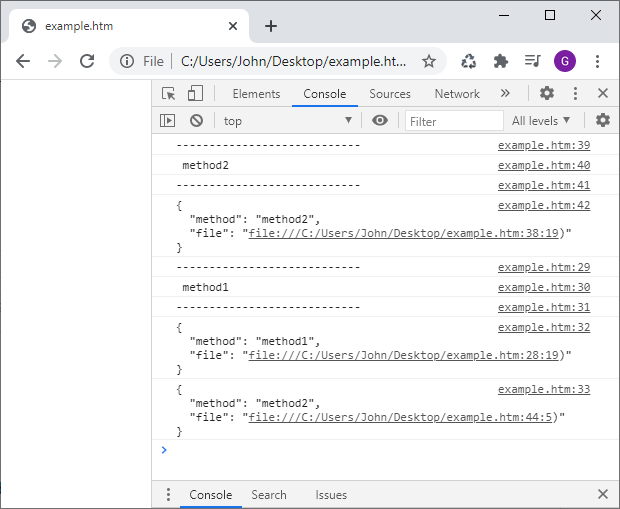