EN
Node.js - PostgreSQL Select last row
0 points
In this article, we would like to show you how to select the last row in the Postgres database from Node.js level.
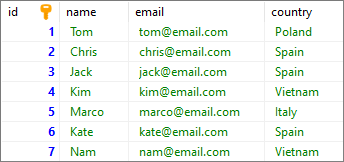
Note: at the end of this article you can find database preparation SQL queries.
xxxxxxxxxx
1
const { Client } = require('pg');
2
3
const client = new Client({
4
host: '127.0.0.1',
5
user: 'my_username',
6
database: 'my_database',
7
password: 'my_password',
8
port: 5432,
9
});
10
11
const fetchLastRows = async (count) => {
12
const query = `
13
SELECT * FROM "users"
14
ORDER BY "id" DESC
15
LIMIT $1;
16
`;
17
await client.connect(); // creates connection
18
try {
19
const { rows } = await client.query(query, [count]); // sends query
20
return rows;
21
} finally {
22
await client.end(); // closes connection
23
}
24
};
25
26
fetchLastRows(1) // get only 1 row from the end of the table
27
.then(result => console.table(result))
28
.catch(error => console.error(error.stack));
Result:
xxxxxxxxxx
1
┌─────────┬────┬───────┬─────────────────┬───────────┐
2
│ (index) │ id │ name │ email │ country │
3
├─────────┼────┼───────┼─────────────────┼───────────┤
4
│ 0 │ 7 │ 'Nam' │ 'nam@email.com' │ 'Vietnam' │
5
└─────────┴────┴───────┴─────────────────┴───────────┘
create_tables.sql
file:
xxxxxxxxxx
1
CREATE TABLE "users" (
2
"id" SERIAL,
3
"name" VARCHAR(100) NOT NULL,
4
"email" VARCHAR(100) NOT NULL,
5
"country" VARCHAR(15) NOT NULL,
6
PRIMARY KEY ("id")
7
);
insert_data.sql
file:
xxxxxxxxxx
1
INSERT INTO "users"
2
("name", "email", "country")
3
VALUES
4
('Tom', 'tom@email.com', 'Poland'),
5
('Chris', 'chris@email.com', 'Spain'),
6
('Jack', 'jack@email.com', 'Spain'),
7
('Kim', 'kim@email.com', 'Vietnam'),
8
('Marco', 'marco@email.com', 'Italy'),
9
('Kate', 'kate@email.com', 'Spain'),
10
('Nam', 'nam@email.com', 'Vietnam');
Native SQL query (used in the above example):
xxxxxxxxxx
1
SELECT * FROM "users"
2
ORDER BY "id" DESC
3
LIMIT 1;