EN
Spring Boot 2 - broadcast messages to each application instance using RabbitMQ
3 points
In this article, we would like to show you how to create Spring Boot 2 application that broadcasts messages to each application instance that uses RabbitMQ.
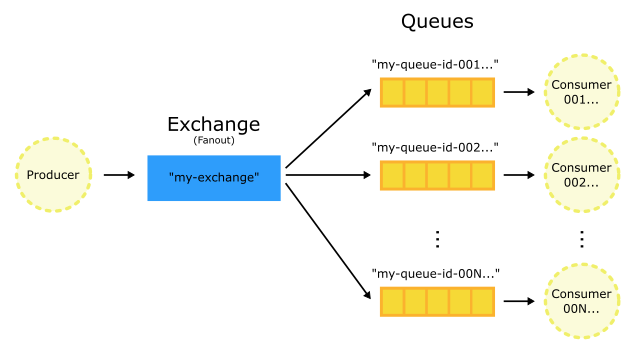
In this section, we can find a simplified solution that realizes broadcasting.
Hint: the article extends project in this article.
ProducerConfig.java
file:
xxxxxxxxxx
1
package com.example.demo;
2
3
import org.springframework.amqp.core.FanoutExchange;
4
import org.springframework.context.annotation.Bean;
5
import org.springframework.context.annotation.Configuration;
6
7
8
public class ProducerConfig {
9
10
11
public FanoutExchange broadcastExchange() {
12
return new FanoutExchange("my-exchange");
13
}
14
}
ProducerController.java
file:
xxxxxxxxxx
1
package com.example.demo;
2
3
import org.springframework.beans.factory.annotation.Autowired;
4
import org.springframework.stereotype.Controller;
5
import org.springframework.web.bind.annotation.RequestMapping;
6
import org.springframework.web.bind.annotation.ResponseBody;
7
8
9
public class ProducerController {
10
11
12
private ProducerService producerService;
13
14
"/test") (
15
16
public void test() {
17
this.producerService.broadcastMessage("Testing message...");
18
}
19
}
ProducerService.java
file:
xxxxxxxxxx
1
package com.example.demo;
2
3
import org.springframework.amqp.rabbit.core.RabbitTemplate;
4
import org.springframework.beans.factory.annotation.Autowired;
5
import org.springframework.stereotype.Service;
6
7
8
public class ProducerService {
9
10
11
private RabbitTemplate template;
12
13
public void broadcastMessage(String message) {
14
this.template.convertAndSend("my-exchange", "", message); // broadcasts string message to each my-queue-* via my-exchange
15
}
16
}
ConsumerConfig.java
file:
xxxxxxxxxx
1
package com.example.demo;
2
3
import org.springframework.amqp.core.Binding;
4
import org.springframework.amqp.core.BindingBuilder;
5
import org.springframework.amqp.core.FanoutExchange;
6
import org.springframework.amqp.core.Queue;
7
import org.springframework.amqp.rabbit.annotation.EnableRabbit;
8
import org.springframework.amqp.rabbit.connection.ConnectionFactory;
9
import org.springframework.amqp.rabbit.listener.SimpleMessageListenerContainer;
10
import org.springframework.amqp.rabbit.listener.adapter.MessageListenerAdapter;
11
import org.springframework.context.annotation.Bean;
12
import org.springframework.context.annotation.Configuration;
13
14
import java.util.UUID;
15
16
17
18
public class ConsumerConfig {
19
20
21
public String instanceId() {
22
return "id-" + UUID.randomUUID();
23
}
24
25
26
public FanoutExchange broadcastExchange() {
27
return new FanoutExchange("my-exchange");
28
}
29
30
31
public Queue instanceQueue(String instanceId) {
32
return new Queue("my-queue-" + instanceId, true);
33
}
34
35
36
public Binding instanceBinding(Queue instanceQueue, FanoutExchange broadcastExchange) {
37
return BindingBuilder.bind(instanceQueue).to(broadcastExchange);
38
}
39
40
41
public SimpleMessageListenerContainer container(String instanceId, ConnectionFactory connectionFactory, MessageListenerAdapter listenerAdapter) {
42
SimpleMessageListenerContainer container = new SimpleMessageListenerContainer();
43
container.setConnectionFactory(connectionFactory);
44
container.setQueueNames("my-queue-" + instanceId);
45
container.setMessageListener(listenerAdapter);
46
return container;
47
}
48
}
ConsumerService.java
file:
xxxxxxxxxx
1
package com.example.demo;
2
3
import org.springframework.amqp.rabbit.listener.adapter.MessageListenerAdapter;
4
import org.springframework.context.annotation.Bean;
5
import org.springframework.stereotype.Service;
6
7
8
public class ConsumerService {
9
10
11
public MessageListenerAdapter listenerAdapter(ConsumerService consumerService) {
12
return new MessageListenerAdapter(consumerService, "handleMessage");
13
}
14
15
public void handleMessage(String message) {
16
System.out.println("Message: " + message);
17
}
18
}