EN
JavaScript - upload file with jQuery
9 points
In this article, we would like to show you how to upload files with jQuery in JavaScript.
ajax.htm
file:
xxxxxxxxxx
1
2
<html lang="en">
3
<head>
4
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.4.1/jquery.min.js"></script>
5
</head>
6
<body>
7
<form id="form" method="POST" onsubmit="sendForm(); return false;">
8
<input type="file" id="file" />
9
<input type="submit" value="Upload" />
10
</form>
11
<pre id="progress"></pre>
12
<script>
13
14
var file = document.getElementById('file');
15
var progress = document.getElementById('progress');
16
17
function sendForm() {
18
var data = new FormData();
19
20
var files = file.files;
21
22
for (var i = 0; i < files.length; ++i) {
23
data.append('file', files[i]);
24
}
25
26
$.ajax({
27
type: 'POST',
28
url: '/backend.php',
29
data: data,
30
contentType: false,
31
processData: false,
32
xhr: function () {
33
var xhr = $.ajaxSettings.xhr();
34
35
if (xhr.upload) {
36
xhr.upload.onprogress = function(e) {
37
var tmp = Math.round(100.0 * (e.loaded / e.total));
38
39
progress.innerText = 'Uploaded ' + tmp + '%';
40
};
41
}
42
43
return xhr;
44
},
45
success: function (data) {
46
file.value = '';
47
48
progress.innerText = data;
49
},
50
error: function(error) {
51
progress.innerText = 'Error: ' + error.status;
52
}
53
});
54
}
55
56
</script>
57
</body>
58
</html>
backend.php
file:
xxxxxxxxxx
1
2
3
define('DST_DIR', 'C:\\wamp64\\www\\uploaded_files\\');
4
5
if($_SERVER['REQUEST_METHOD'] === 'POST')
6
{
7
if(isset($_FILES['file']))
8
{
9
$file = $_FILES['file'];
10
11
$src_path = $file['tmp_name'];
12
$dst_path = DST_DIR . $file['name'];
13
14
move_uploaded_file($src_path, $dst_path);
15
16
echo "File uploaded successfully.";
17
}
18
else
19
echo "File does not exist.";
20
}
21
else
22
echo "Unsupported request method.";
Notes:
ajax.htm
andbackend.php
should be both places on same php server- directory path
C:\wamp64\www\uploaded_files\
should be changed to own path - do not forget to put backslash at the end of path and have permission for files writing there
Result:
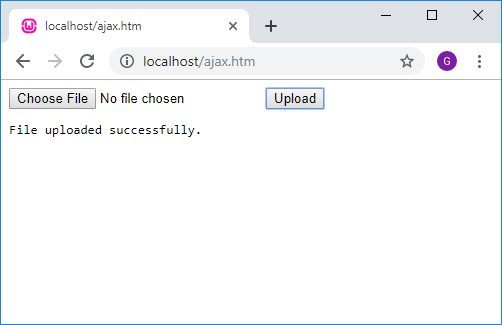