EN
JavaScript - ajax request
1
points
In this article, we would like to show you how to make AJAX requests in JavaScript.
1. Pure JavaScript (Vanilla JS) AJAX request with XMLHttpRequest
object example
Browser site GET and POST methods example:
<!doctype html>
<html lang="en">
<body>
<script>
var xhr = new XMLHttpRequest();
xhr.onreadystatechange = function () {
// if request done
if (xhr.readyState == XMLHttpRequest.DONE) {
// if 200 http status received
if (xhr.status == 200)
// server response as text
document.body.innerText = 'Response: ' + xhr.responseText;
else
// error status
document.body.innerText = 'Error: ' + xhr.status;
}
};
// initialize or re-initialize GET request
xhr.open('GET', '/echo', true);
// send request without any data
xhr.send(null);
/*
// POST method example:
var data = 'This is my data...';
// initialize or re-initialize GET request
xhr.open('POST', '/echo', true);
// send request with data
xhr.send(data);
*/
</script>
</body>
</html>
SpringMVC server site GET and POST methods example:
package com.dirask.ckeditor4.remake.spring.boot.controller;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.*;
@Controller
public class EchoController {
@RequestMapping(value = "/echo", method = RequestMethod.GET)
@ResponseBody
public String makeGetEcho()
{
return "Echo...";
}
/*
// Alternative POST method example:
@RequestMapping(value = "/echo", method = RequestMethod.POST)
@ResponseBody
public String makePostEcho(@RequestBody String data)
{
return data;
}
*/
}
Note: run both logics on same server.
Result:
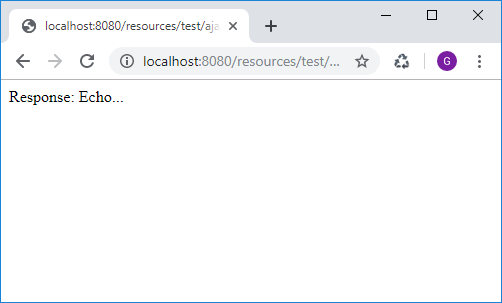
2. jQuery AJAX request exampleÂ
Browser site GET and POST methods example:
<!doctype html>
<html lang="en">
<head>
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.4.1/jquery.min.js"></script>
</head>
<body>
<script>
$.ajax({
type: 'GET',
url: '/echo',
success: function (data) {
document.body.innerText = 'Response: ' + data;
},
error: function(jqXHR) {
document.body.innerText = 'Error: ' + jqXHR.status;
}
});
/*
// POST method example:
$.ajax({
type: 'POST',
url: '/echo',
data: 'This is my data...',
success: function (data) {
document.body.innerText = 'Response: ' + data;
},
error: function(jqXHR) {
document.body.innerText = 'Error: ' + jqXHR.status;
}
});
// or
$.get('/echo')
.done(function(data) {
document.body.innerText = 'Response: ' + data;
})
.fail(function(jqXHR) {
document.body.innerText = 'Error: ' + jqXHR.status;
});
// or
$.post('/echo', 'This is my data...')
.done(function(data) {
document.body.innerText = 'Response: ' + data;
})
.fail(function(jqXHR) {
document.body.innerText = 'Error: ' + jqXHR.status;
});
*/
</script>
</body>
</html>
SpringMVC server site GET and POST methods example:
package com.dirask.ckeditor4.remake.spring.boot.controller;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.*;
@Controller
public class EchoController {
@RequestMapping(value = "/echo", method = RequestMethod.GET)
@ResponseBody
public String makeGetEcho()
{
return "Echo...";
}
/*
// Alternative POST method example:
@RequestMapping(value = "/echo", method = RequestMethod.POST)
@ResponseBody
public String makePostEcho(@RequestBody String data)
{
return data;
}
*/
}
Note: run both logics on same server.
Result:
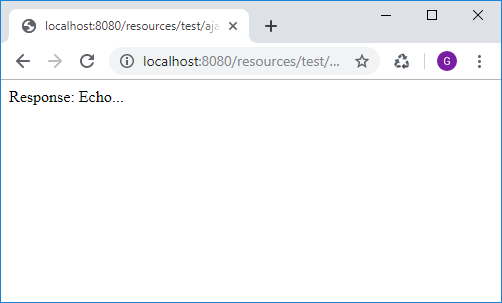
3. fetch
method example
Browser site GET and POST methods example:
<!doctype html>
<html lang="en">
<body>
<script>
fetch('/echo')
.then(data => {
data.text()
.then(text => {
document.body.innerText = 'Response: ' + text;
});
})
.catch(error => {
console.log('Error:', error);
});
/*
// POST method example:
var config = {
method: 'POST',
body: 'This is my data...'
};
fetch('/echo', config)
.then(data => {
data.text()
.then(text => {
document.body.innerText = 'Response: ' + text;
});
})
.catch(error => {
console.log('Error:', error);
});
*/
</script>
</body>
</html>
SpringMVC server site GET and POST methods example:
package com.dirask.ckeditor4.remake.spring.boot.controller;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.*;
@Controller
public class EchoController {
@RequestMapping(value = "/echo", method = RequestMethod.GET)
@ResponseBody
public String makeGetEcho()
{
return "Echo...";
}
/*
// Alternative POST method example:
@RequestMapping(value = "/echo", method = RequestMethod.POST)
@ResponseBody
public String makePostEcho(@RequestBody String data)
{
return data;
}
*/
}
Note: run both logics on same server.
Result:
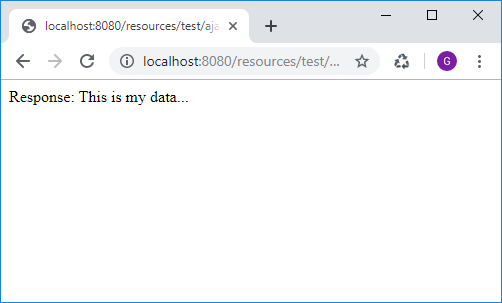
4. fetch
 method with ES7 async/await example
Browser site GET and POST methods example:
<!doctype html>
<html lang="en">
<body>
<script>
async function doRequest() {
/*
// Uncomment this source code for POST request method
var config = {
method: 'POST',
body: 'This is my data...'
};
*/
try {
const response = await fetch('/echo'/*, config*/);
const text = await response.text();
document.body.innerText = 'Response: ' + text;
} catch (error) {
console.log('Error:', error);
}
}
doRequest();
</script>
</body>
</html>
SpringMVC server site GET and POST methods example:
package com.dirask.ckeditor4.remake.spring.boot.controller;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.*;
@Controller
public class EchoController {
@RequestMapping(value = "/echo", method = RequestMethod.GET)
@ResponseBody
public String makeGetEcho()
{
return "Echo...";
}
/*
// Alternative POST method example:
@RequestMapping(value = "/echo", method = RequestMethod.POST)
@ResponseBody
public String makePostEcho(@RequestBody String data)
{
return data;
}
*/
}
Note: run both logics on same server.
Result:
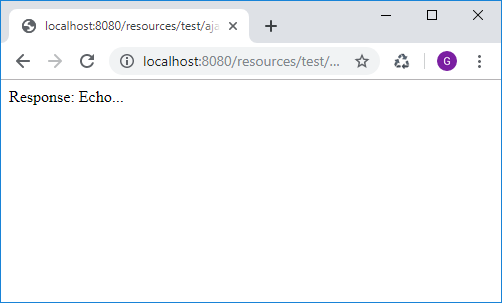