EN
TypeScript - binary search algorithm example
0 points
In this short article, we would like to show the main idea of the binary search algorithm providing example implementation in TypeScript.
Binary search is a fast search algorithm with run-time complexity O(log n)
. This algorithm requires working on sorted data with random access to each element.
The main idea of the binary search algorithm is to:
- find middle point index in actual range with the formula:
const point: number = Math.ceil((index + limit) / 2);
Notes:- the actual range has been marked with a blue dashed border on the below image,
- middle point index has been marked with a black arrow on the below image,
- in the beginning, the actual range contains all array elements (
index=0
,limit=array.length-1
), - the middle point index is used to split the searching problem into 2 smaller ranges - only if it is necessary for the next steps,
- if the middle point value is equal to the expected value, the algorithm returns the middle point index,
Notes:- expected value has been marked with a yellow rounded rectangle on the below image,
- if the middle point value is not equal to the expected value, the range is split into left or right ranges with an excluded middle point, a new range that is likely to contain the expected value is selected as an actual range and we go back to 1. point (we work all-time on sorted data).
Notes:- if the expected value is smaller than the middle point value we select left range,
- if the expected value is bigger than the middle point value we select right range,
Algorithm iterations:
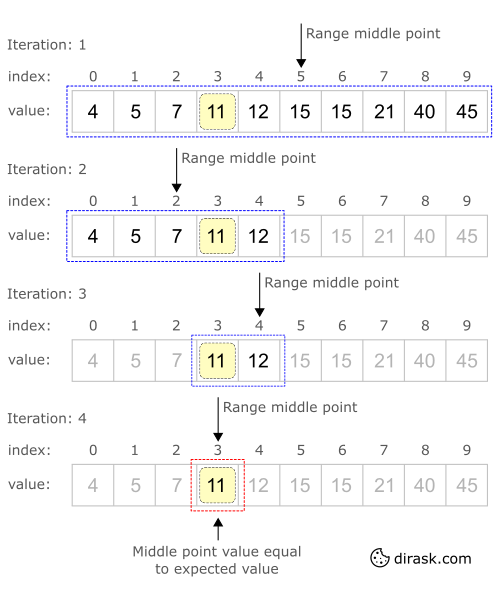
xxxxxxxxxx
1
const searchIndex = (array: number[], value: number): number => {
2
let index = 0;
3
let limit = array.length - 1;
4
while (index <= limit) {
5
const point = Math.ceil((index + limit) / 2);
6
const entry = array[point];
7
if (value > entry) {
8
index = point + 1;
9
continue;
10
}
11
if (value < entry) {
12
limit = point - 1;
13
continue;
14
}
15
return point; // value == entry
16
}
17
return -1;
18
};
19
20
21
// Usage example:
22
23
// for this implementation array must be sorted from smallest to biggest!
24
const array: number[] = [4, 5, 7, 11, 12, 15, 15, 21, 40, 45];
25
const index: number = searchIndex(array, 11); // we want to find index for 11
26
27
console.log(index); // 3
Output:
xxxxxxxxxx
1
3
xxxxxxxxxx
1
const searchIndex = (array: number[], value: number): number => {
2
const split = (index: number, limit: number) => {
3
if (index > limit) {
4
return -1;
5
}
6
const point = Math.ceil((limit + index) / 2);
7
const entry = array[point];
8
if (value < entry) {
9
return split(index, point - 1);
10
}
11
if (value > entry) {
12
return split(point + 1, limit);
13
}
14
return point; // value == entry
15
};
16
return split(0, array.length - 1);
17
};
18
19
20
// Usage example:
21
22
// for this implementation array must be sorted from smallest to biggest!
23
const array: number[] = [4, 5, 7, 11, 12, 15, 15, 21, 40, 45];
24
const index: number = searchIndex(array, 11); // we want to find index for 11
25
26
console.log(index); // 3
Output:
xxxxxxxxxx
1
3