EN
TypeScript - ReturnType<> with generic function
6 points
In this short aritcle we would like to show you how to get result type from generic function in TypeScript.
Problem description:
typeof
operator was created to work with variables in runtime mode
e. g.typeof myFunction
,- when
myFunction
is generic it is not posible to dotypeof myFunction<Type>
operation, becausemyFunction<Type>
doesnt exists in the application memory - check below example to see some trick.
Quick solution:
xxxxxxxxxx
1
// ----------------------------------------------------------------------------
2
3
// Genric function definition:
4
5
const createCollection = <T extends unknown> (users: T[]) => {
6
return {
7
getUser: (index: number): T | null => {
8
return users[index] ?? null;
9
}
10
};
11
};
12
13
// ----------------------------------------------------------------------------
14
15
// Return type extracting:
16
17
class Wrapper<T extends unknown> {
18
//HINT: do not forget to match arguments to your function
19
mediate = (args: any[]) => createCollection<T>(args);
20
}
21
22
type GenericCollectionReturnType<T extends unknown> = ReturnType<Wrapper<T>['mediate']>;
23
24
// ----------------------------------------------------------------------------
25
26
// Type usage example:
27
28
const users: GenericCollectionReturnType<string> = createCollection('John', 'Kate');
29
30
console.log(users.getUser(0)); // John
31
console.log(users.getUser(1)); // Kate
Screenshot:
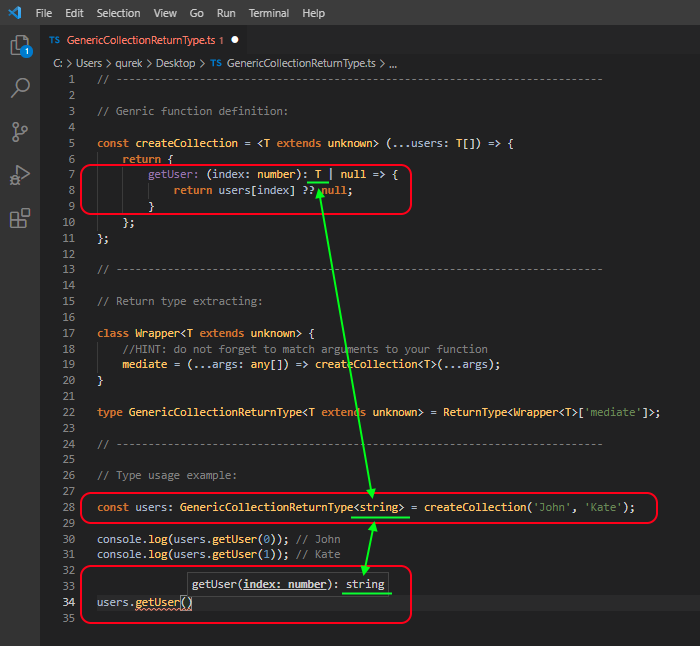