EN
React - how to move element to front using z-index CSS property
3 points
In this article we would like to show you how to move element to front using zIndex
style property (z-index
property equivalent in pure CSS).
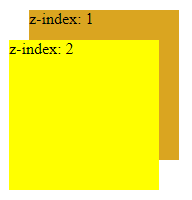
Below example shows two components with different styles:
Component1
withzIndex: '1'
,Component2
withzIndex: '2'
.
An element with greater zIndex
is always in front of an element with a lower zIndex
value, so in our case Component2
(zIndex: '2'
) covers Component1
(zIndex: '1'
).
xxxxxxxxxx
1
// Note: Uncomment import lines in you project.
2
3
// import React from react';
4
// import ReactDOM from 'react-dom';
5
6
const Component1 = () => {
7
const style = {
8
position: 'absolute', // <------ required
9
left: '30px', // <------ also may be required
10
top: '10px', // <------ also may be required
11
background: 'goldenrod',
12
width: '100px',
13
height: '100px',
14
zIndex: '1' // <------ required
15
};
16
return (
17
<div style={style}>
18
z-index: 1
19
</div>
20
);
21
};
22
23
const Component2 = () => {
24
const style = {
25
position: 'absolute', // <------ required
26
left: '10px', // <------ also may be required
27
top: '40px', // <------ also may be required
28
background: 'yellow',
29
width: '100px',
30
height: '100px',
31
zIndex: '2' // <------ required
32
};
33
return (
34
<div style={style}>
35
z-index: 2
36
</div>
37
);
38
};
39
40
const App = () => {
41
const style = {
42
height: '120px'
43
};
44
return (
45
<div style={style}>
46
<Component2 /> {/* <------ will be displayed over component 1 */}
47
<Component1 /> {/* <------ will be displayed under component 2 */}
48
</div>
49
);
50
};
51
52
const root = document.querySelector('#root');
53
ReactDOM.render(<App />, root);
Note:
zIndex
only works when element has position set to:fixed
,absolute
,relative
orsticky
.