EN
React - create HTML comment (<!-- comment -->)
7
points
In this short article, we would like to show how to create HTML comment from JSX syntax in React.
Quick solution:
const HtmlComment = ({text}) => {
const html = `<!-- ${text} -->`;
const callback = (instance) => {
if (instance) {
instance.outerHTML = html;
}
};
return (<script ref={callback} type="text/comment" dangerouslySetInnerHTML={{__html: html}} />);
};
// Usage: <HtmlComment text="Some HTML comment here ..." />
Ā
Practical examples
1. Reference based solution
In this section, you can find solution that injects comment using React referenceĀ andĀ outerHTML
Ā DOM property.
Advantages:
- creates pure comment node
Disadvantages:
- doesn'tĀ workĀ in SSR mode (server returns just empty
<script type="text/placeholder"></script>
element that in the next step isĀ rendered in the web browser as comment node), - it is required to escape special characters by self from
text
prop.
import React, {useRef, useEffect} from 'react';
const HtmlComment = ({text}) => {
const ref = useRef();
useEffect(() => {
ref.current.outerHTML = `<!--${text}-->`;
}, [text]);
return (<script ref={ref} type="text/placeholder" />);
};
// Usage example:
const App = () => {
return (
<div>
<HtmlComment text="Some HTML comment here ..." />
</div>
);
};
export default App;
Generated source code preview:
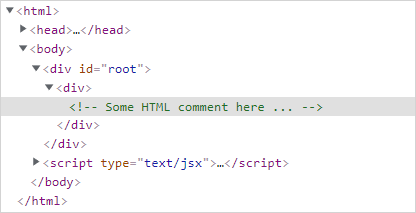
Ā
2. Property based solution
In this section, you can find solution that injects comment usingĀ dangerouslySetInnerHTML
React property.
Advantages:
- works in SSR mode
Disadvantages:
- uses additional wrapping HTML elementĀ (comment is located insideĀ
<span></span>
element), - it is required to escape special characters by self from
text
prop.
import React from 'react';
const HtmlComment = ({text}) => {
return (<span dangerouslySetInnerHTML={{__html: `<!-- ${text} -->`}} />);
};
// Usage example:
const App = () => {
return (
<div>
<HtmlComment text="Some HTML comment here ..." />
</div>
);
};
export default App;
Generated source code preview:
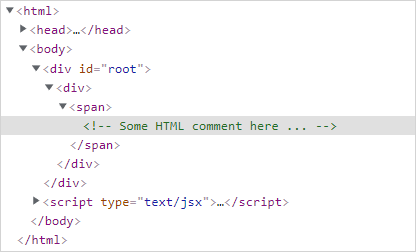
Ā
3. Mixed solution
In this section, you can find solution that injects comment togather usingĀ dangerouslySetInnerHTML
React property and React references andĀ outerHTML
Ā DOM property.
Advantages:
- works in SSR mode,
- finally creates pure comment node.
Disadvantages:
- comment returned from server differs than rendered in the web browser what finally is accepted.
- it is required to escape special characters by self from
text
prop.
import React from 'react';
const HtmlComment = ({text}) => {
const html = `<!-- ${text} -->`;
const callback = (instance) => {
if (instance) {
instance.outerHTML = html;
}
};
return (<script ref={callback} type="text/comment" dangerouslySetInnerHTML={{__html: html}} />);
};
// Usage example:
const App = () => {
return (
<div>
<HtmlComment text="Some HTML comment here ..." />
</div>
);
};
export default App;
Ā