EN
Node.js - read JSON files
3
points
In this article, we would like to show you how to read JSON files in Node.js.
Quick solution:
const fs = require('fs');
try {
const fileJson = fs.readFileSync('/path/to/file.json', 'utf-8');
const fileData = JSON.parse(fileJson);
console.log(fileData);
} catch (ex) {
console.error(ex);
}
Example preview:
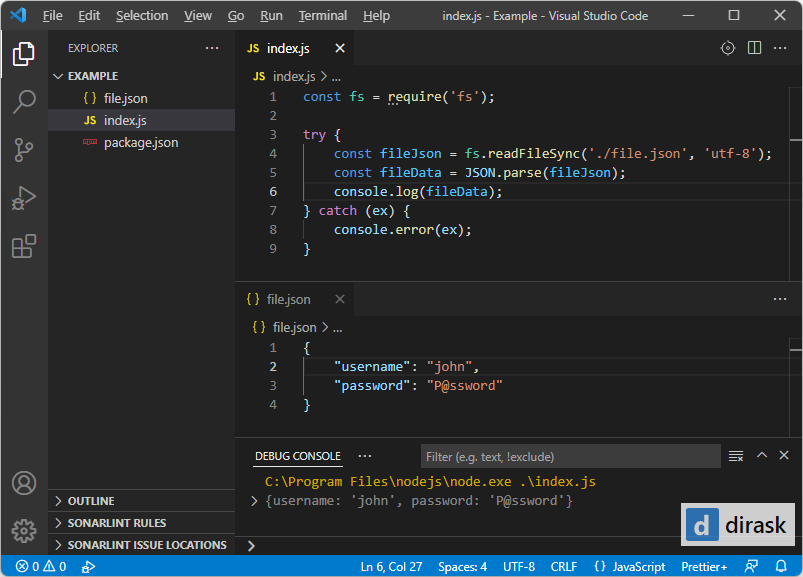
In the below, we present 3 methods to read JSON files:
- synchronously,
- asynchronously with callbacks,
- asynchronously with
async
/await
keywords.
Synchronously
Is recommended to use synchronous operations when we want to get better performance - e.g. Node JS-based sequential scripts run under the operating system.
const fs = require('fs');
const readJsonFile = (path) => {
const fileJson = fs.readFileSync(path, 'utf-8');
const fileData = JSON.parse(fileJson);
return fileData;
};
// Usage example:
const fileData = readJsonFile('/path/to/file.json');
console.log(fileData);
Asynchronously with callback
This approach is commonly used in Node.js web applications.
const fs = require('fs');
const readJsonFile = (path, callback) => {
fs.readFile(path, 'utf-8', (error, fileJson) => {
if (error) {
callback(error, undefined);
} else {
try {
const fileData = JSON.parse(fileJson);
callback(undefined, fileData);
} catch (ex) {
callback(ex, undefined);
}
}
});
};
// Usage example:
readJsonFile('/path/to/file.json', (error, fileData) => {
if (error) {
console.log(error);
} else {
console.log(fileData);
}
});
Asynchronously with async/await
The main advantage of this approach is source code readability.
const { readFile } = require('fs/promises');
const readJsonFile = async (path) => {
const jsonData = await readFile(path, 'utf-8');
const parsedData = JSON.parse(jsonData);
return parsedData;
};
// Usage example 1:
const someMethod = async () => {
try {
const fileData = await readJsonFile('/path/to/file.json');
console.log(fileData);
} catch (ex) {
console.error(ex);
}
};
someMethod();
// Usage example 2:
readJsonFile('/path/to/file.json')
.then((fileData) => console.log(fileData))
.catch((ex) => console.error(ex));