EN
Node.js - create directory using path
0
points
In this article, we would like to show you how to create directory / folder using fs.mkdirSync
method in Node.js.
Quick solution:
const fs = require('fs');
const directoryPath = './newFolder';
if (!fs.existsSync(directoryPath)) {
fs.mkdirSync(directoryPath);
}
Practical example
In this example, we create a new folder under the given path.
const fs = require('fs');
const directoryPath = './newFolder';
if (!fs.existsSync(directoryPath)) {
fs.mkdirSync(directoryPath);
} else {
console.log('File or directory already exist!');
}
Recursive version
if we want to create folders that are nested, we have to set the recursive flag in the mkdirSync()
method.
const fs = require('fs');
const directoryPath = 'C:/projects/example/newFolder';
if (!fs.existsSync(directoryPath)) {
fs.mkdirSync(directoryPath, { recursive: true });
} else {
console.log('File or directory already exist!');
}
Result:
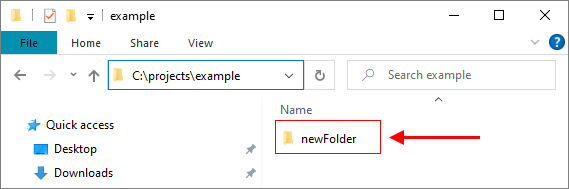