EN
Next.js - create dynamic routes
0
points
In this article, we would like to show you how to create dynamic routes in Next.js.
Practical example
In this example, we present how to display the details of the product with given id
, when the user navigates to the /product
route followed by the id
of the product.
Example URL:
http://localhost:3000/product/1
Result:
The route will return the details about the product with id
= 1
.
Project structure
In order to create dynamic routes, need to create the following project structure:
- inside
pages/
directory, create the separateproduct/
folder for the products, - inside
product/
folder, create[id].js
file - by wraping theid
with square brackets we create a dynamic route, where we can passid
of the individual products. product/index.js
file, will be mapped to the main/product
route,
/next.js-project/
├─ next/
├─ node_modules/
└─ pages/
├─ api/
└─ product/
├─ index.js
└─ [id].js
Example components
product/index.js
:
function Product() {
return <h1>Product main page</h1>;
}
export default Product;
product/[id].js
:
import { useRouter } from 'next/router';
function ProductDetails() {
const router = useRouter();
const id = router.query.id; // gets id query parameter
return <h1>Product {id} details</h1>;
}
export default ProductDetails;
Web browser preview
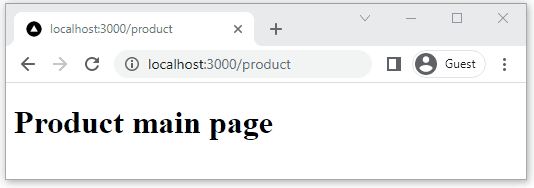