EN
JavaScript - send messages between windows with BroadcastChannel
8
points
In this article, we would like to show you how we can communicate between different browser tabs.
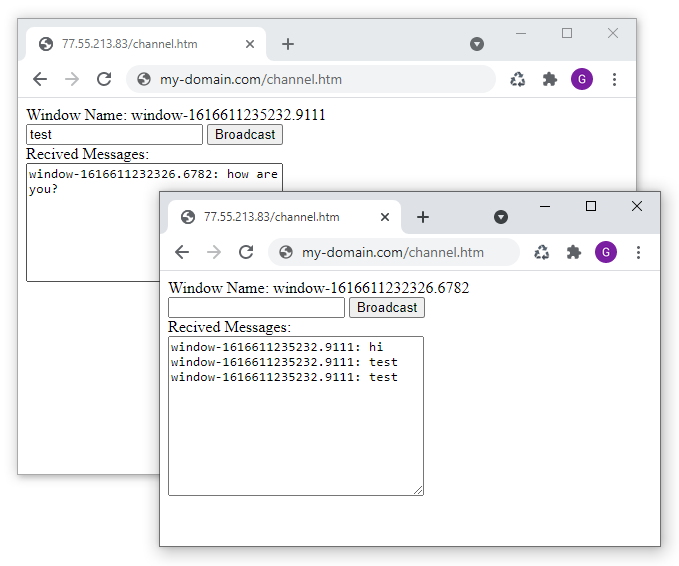
Quick solution:
const broadcastChannel = new BroadcastChannel('my-channel-name');
broadcastChannel.addEventListener('message', event => {
const message = event.data;
...
});
const broadcastMessage = (message) => {
broadcastChannel.postMessage(message);
};
BroadcastChannel
allows communication between tabs, frames, iframes and windows. The connection is made in the browser and doesn't need a backend.
The message is broadcast using the postMessage()
method and the recipients are windows that listen through the addEventListener.
Note:
Open this post in two or more tabs/windows, run example and send messages.
Practical example:
// ONLINE-RUNNER:browser;
<html>
<body>
<div id="window-name">Window name: null</div>
<div>
<input id="message-text" />
<button onclick="handleClick()">Broadcast</button>
</div>
<div>
<div>Recived messages:</div>
<textarea id="recived-messages" style="width: 260px; height: 200px"></textarea>
</div>
<script>
const windowNameElement = document.querySelector('#window-name');
const messageTextElement = document.querySelector('#message-text');
const recivedMessagesElement = document.querySelector('#recived-messages');
// ---------------------------------
const windowName = `window-${Date.now()}${Math.random()}`; // ~~ unique window name
const channelName = 'my-notifications'; // any name here
const broadcastChannel = new BroadcastChannel(channelName);
broadcastChannel.addEventListener('message', event => {
const message = event.data;
recivedMessagesElement.value += `${message.windowName}: ${message.windowMessage}\n`;
});
const broadcastMessage = (windowMessage) => {
const message = {
windowName: windowName,
windowMessage: windowMessage
};
broadcastChannel.postMessage(message);
};
// ---------------------------------
windowNameElement.innerText = `Window name: ${windowName}`;
const handleClick = (windowMessage) => {
broadcastMessage(messageTextElement.value);
messageTextElement.value = '';
};
</script>
</body>
</html>
Note:
BroadcastChannel
interface is not supported in Internet Explorer, Safari and older browsers, so use with caution.