EN
JavaScript - preview selected image in input type="file"
6 points
In this short article, we would like to show how using JavaScript, display preview for input file element when we select image file.
Simple preview:
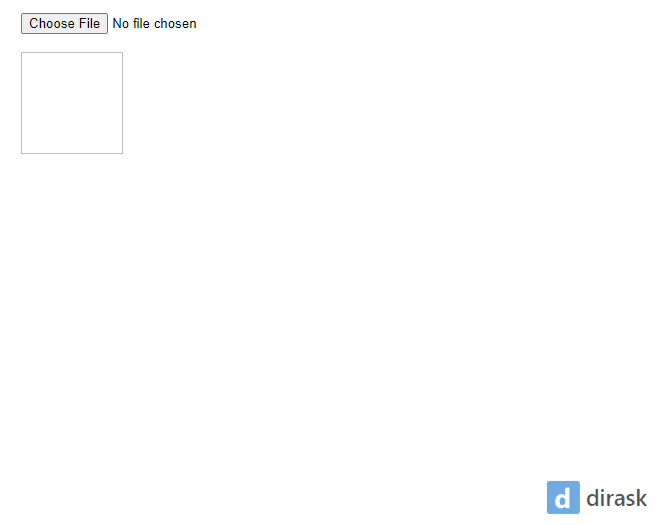
Practical example:
xxxxxxxxxx
1
2
<html>
3
<head>
4
<style>
5
6
img.image {
7
border: 1px solid silver;
8
width: 100px;
9
height: 100px;
10
}
11
12
</style>
13
</head>
14
<body>
15
<input class="file" id="my-file" type="file" accept="image/*" />
16
<br /><br />
17
<img class="image" id="my-image" src="data:image/gif;base64,R0lGODlhAQABAIAAAP///wAAACH5BAEAAAAALAAAAAABAAEAAAICRAEAOw==" alt="My image" />
18
<script>
19
20
var DEFAULT_IMAGE = 'data:image/gif;base64,R0lGODlhAQABAIAAAP///wAAACH5BAEAAAAALAAAAAABAAEAAAICRAEAOw==';
21
22
var hImage = document.querySelector('#my-image');
23
var hFile = document.querySelector('#my-file');
24
25
var reader = new FileReader();
26
27
reader.onload = function (e) {
28
hImage.src = e.target.result;
29
};
30
31
hFile.onchange = function() {
32
var files = hFile.files;
33
if (files.length > 0) {
34
reader.readAsDataURL(files[0]);
35
} else {
36
hImage.src = DEFAULT_IMAGE;
37
}
38
};
39
40
</script>
41
</body>
42
</html>