EN
JavaScript - ini file with CodeMirror 5
14
points
In this article, we would like to show how to highlit *.ini file syntax using CodeMirror in JavaScript.
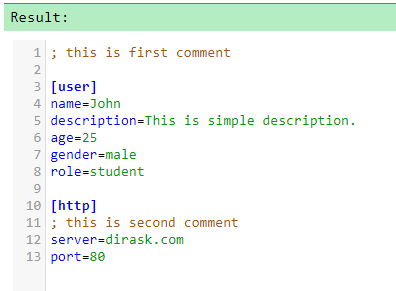
Note: to highlight
*.ini
files it is necessary to setmode
totext/x-ini
.
1. INI file set from variable
The example in this section shows how to indicate the type of content for CodeMirror.
// ONLINE-RUNNER:browser;
<!doctype html>
<html>
<head>
<link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/codemirror/5.48.4/codemirror.min.css" />
<script type="text/javascript" src="https://cdnjs.cloudflare.com/ajax/libs/codemirror/5.48.4/codemirror.min.js"></script>
<script type="text/javascript" src="https://cdnjs.cloudflare.com/ajax/libs/codemirror/5.48.4/mode/properties/properties.min.js"></script>
</head>
<body>
<div id="placeholder"></div>
<script>
var placeholder = document.getElementById('placeholder');
var editor = CodeMirror(placeholder, {
mode: 'text/x-ini',
readOnly: false,
lineNumbers: true,
lineWrapping: false
});
var text = '; this is first comment\n' +
'\n' +
'[user]\n' +
'name=John\n' +
'description=This is simple description.\n' +
'age=25\n' +
'gender=male\n' +
'role=student\n' +
'\n' +
'[http]\n' +
'; this is second comment\n' +
'server=dirask.com\n' +
'port=80';
editor.setValue(text);
</script>
</body>
</html>
2. INI file syntax highlighting detected automatically and set from variable
The example in this section shows how to detect ini file automatically.
// ONLINE-RUNNER:browser;
<!doctype html>
<html>
<head>
<link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/codemirror/5.48.4/codemirror.min.css" />
<script type="text/javascript" src="https://cdnjs.cloudflare.com/ajax/libs/codemirror/5.48.4/codemirror.min.js"></script>
<script type="text/javascript" src="https://cdnjs.cloudflare.com/ajax/libs/codemirror/5.48.4/mode/properties/properties.min.js"></script>
</head>
<body>
<div id="placeholder"></div>
<script>
var placeholder = document.getElementById('placeholder');
// CodeMirror detects automatically file type
var editor = CodeMirror(placeholder, {
readOnly: false,
lineNumbers: true,
lineWrapping: false
});
var text = '; this is first comment\n' +
'\n' +
'[user]\n' +
'name=John\n' +
'description=This is simple description.\n' +
'age=25\n' +
'gender=male\n' +
'role=student\n' +
'\n' +
'[http]\n' +
'; this is second comment\n' +
'server=dirask.com\n' +
'port=80';
editor.setValue(text);
</script>
</body>
</html>
3. INI file syntax highlighting built on textarea
element
The example in this section shows how to indicate the type of content for CodeMirror and replace indicated textarea
element with beautifully highlighted code.
// ONLINE-RUNNER:browser;
<!doctype html>
<html>
<head>
<link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/codemirror/5.48.4/codemirror.min.css" />
<script type="text/javascript" src="https://cdnjs.cloudflare.com/ajax/libs/codemirror/5.48.4/codemirror.min.js"></script>
<script type="text/javascript" src="https://cdnjs.cloudflare.com/ajax/libs/codemirror/5.48.4/mode/properties/properties.min.js"></script>
</head>
<body>
<textarea id="textarea">; this is first comment
[user]
name=John
description=This is simple description.
age=25
gender=male
role=student
[http]
; this is second comment
server=dirask.com
port=80</textarea >
<script>
var textarea= document.getElementById('textarea');
var editor = CodeMirror.fromTextArea(textarea, {
mode: 'text/x-ini'
});
</script>
</body>
</html>
4. INI file syntax highlighting detected automatically and built on textarea
element
The example in this section shows how to detect ini file automatically for CodeMirror and replace indicated textarea
element with beautifully highlighted code.
// ONLINE-RUNNER:browser;
<!doctype html>
<html>
<head>
<link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/codemirror/5.48.4/codemirror.min.css" />
<script type="text/javascript" src="https://cdnjs.cloudflare.com/ajax/libs/codemirror/5.48.4/codemirror.min.js"></script>
<script type="text/javascript" src="https://cdnjs.cloudflare.com/ajax/libs/codemirror/5.48.4/mode/properties/properties.min.js"></script>
</head>
<body>
<textarea id="textarea">; this is first comment
[user]
name=John
description=This is simple description.
age=25
gender=male
role=student
[http]
; this is second comment
server=dirask.com
port=80</textarea >
<script>
var textarea= document.getElementById('textarea');
// CodeMirror detects automatically file type
var editor = CodeMirror.fromTextArea(textarea, {});
</script>
</body>
</html>