JavaScript - how to escape html special characters?
In this article, we would like to show how to escape special HTML characters using JavaScript.
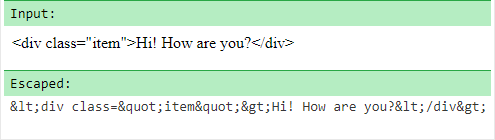
Quick solution (check section 1 to see working in Node.js version):
// ONLINE-RUNNER:browser;
const container = document.createElement('div');
container.innerText = '<div>Some HTML here ...</div>';
const escapedHtml = container.innerHTML;
console.log(escapedHtml); // <div>Some HTML here ...</div>
//
// for solution that works with " and ' characters too
// check '1. Custom function example' section
Custom function example - based on replace
method
In this section presented solution replaces special HTML characters with codes (check characters list here).
Note: this solution works under Node.js.
// ONLINE-RUNNER:browser;
<!doctype html>
<html>
<body>
<script>
var HTMLUtils = new function() {
var rules = [
{ expression: /&/g, replacement: '&' }, // keep this rule at first position
{ expression: /</g, replacement: '<' },
{ expression: />/g, replacement: '>' },
{ expression: /"/g, replacement: '"' },
{ expression: /'/g, replacement: ''' } // or ' or '
// ' is not supported by IE8
// ' is not defined in HTML 4
];
this.escape = function(html) {
var result = html;
for (var i = 0; i < rules.length; ++i) {
var rule = rules[i];
result = result.replace(rule.expression, rule.replacement);
}
return result;
}
};
// Usage example:
var escapedHtml = HTMLUtils.escape('<div class="item">Hi! How are you?</div>');
// Printing in body:
document.body.innerHTML = escapedHtml; // we can display it as text too
// Printing in console:
console.log(escapedHtml); // <div class="item">Hi! How are you?</div>
</script>
</body>
</html>
innerText
property example
By setting the text on the element using innerText
and getting it back with innerHTML
we are able to escape special HTML characters.
Note: by default, this approach doesn't work under Node.js because the DOM is not supported there (required additional libraries).
// ONLINE-RUNNER:browser;
<!doctype html>
<html>
<body>
<div id="container" style="border: 1px solid silver;"></div>
<script>
var container = document.querySelector('#container');
// Printing in body:
container.innerText = '<div class="item">Hi! How are you?</div>';
// Printing in console:
console.log(container.innerHTML); // escaped html
</script>
</body>
</html>
textContent
property example
By setting the text on the element using textContent
and getting it back with innerHTML
we are able to escape special HTML characters.
Note: by default, this approach doesn't work under Node.js because the DOM is not supported there (required additional libraries).
// ONLINE-RUNNER:browser;
<!doctype html>
<html>
<body>
<div id="container" style="border: 1px solid silver;"></div>
<script>
var container = document.querySelector('#container');
// Printing in body:
container.textContent = '<div class="item">Hi! How are you?</div>';
// Printing in console:
console.log(container.innerHTML); // escaped html
</script>
</body>
</html>
Container element-based approach examples
The below examples use the same techniques using stored in the memory element.
3.1. Text node and innerHTML
property-based example
// ONLINE-RUNNER:browser;
<!doctype html>
<html>
<body>
<script>
var escapeHtml = function(html) {
var container = document.createElement('div');
var text = document.createTextNode(html);
container.appendChild(text);
return container.innerHTML;
};
// Usage example:
var escapedHtml = escapeHtml('<div class="item">Hi! How are you?</div>');
// Printing in body:
document.body.innerHTML = escapedHtml; // we can display it as text too
// Printing in console:
console.log(escapedHtml); // <div class="item">Hi! How are you?</div>
</script>
</body>
</html>
3.2. innerText
and innerHTML
properties based example
// ONLINE-RUNNER:browser;
<!doctype html>
<html>
<body>
<script>
var escapeHtml = function(html) {
var container = document.createElement('div');
container.innerText = html;
return container.innerHTML;
};
// Usage example:
var escapedHtml = escapeHtml('<div class="item">Hi! How are you?</div>');
// Printing in body:
document.body.innerHTML = escapedHtml; // we can display it as text too
// Printing in console:
console.log(escapedHtml); // <div class="item">Hi! How are you?</div>
</script>
</body>
</html>