EN
JavaScript - get file upload progress
14 points
In this article we would like to show how to get file upload progress in JavaScript.
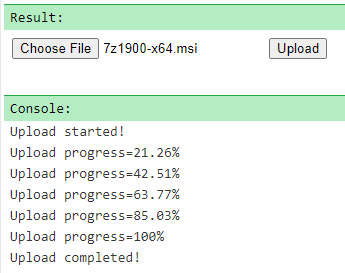
Note: select some e.g.
5 MB
-100 MB
file to see effect.
xxxxxxxxxx
1
<html lang="en">
2
<body>
3
<form id="form">
4
<input name="picture" type="file" />
5
<button id="submit">Upload</button>
6
</form>
7
<script>
8
9
var formElement = document.querySelector('#form');
10
var pictureElement = document.querySelector('#picture');
11
var submitElement = document.querySelector('#submit');
12
var responseElement = document.querySelector('#response');
13
14
submitElement.onclick = function(event) {
15
event.preventDefault();
16
17
var xhr = new XMLHttpRequest();
18
19
if (xhr.upload) {
20
xhr.upload.onprogress = function(event) {
21
if (event.lengthComputable) {
22
// progress in format: 100.00
23
var progress = 0.01 * Math.round(10000 * event.loaded / event.total);
24
console.log('Upload progress=' + progress + '%');
25
}
26
};
27
}
28
29
xhr.onreadystatechange = function () {
30
if (xhr.readyState == XMLHttpRequest.DONE) {
31
if (xhr.status == 200) { // HTTP status 200 received
32
console.log('Upload completed!');
33
console.log('Response: ' + xhr.responseText);
34
} else {
35
console.log('Upload error (status=' + xhr.status + ')');
36
}
37
}
38
};
39
40
var data = new FormData(formElement);
41
42
// or:
43
// var data = {
44
// // some fields here ...
45
// };
46
47
xhr.open('POST', '/gallery/upload', true);
48
xhr.send(data);
49
50
console.log('Upload started!');
51
};
52
53
</script>
54
</body>
55
</html>