EN
JavaScript - convert file paths to tree (multi-level maps)
12 points
In this short article, we would like to show how to convert file paths to tree in JavaScript.
Conversion concept:
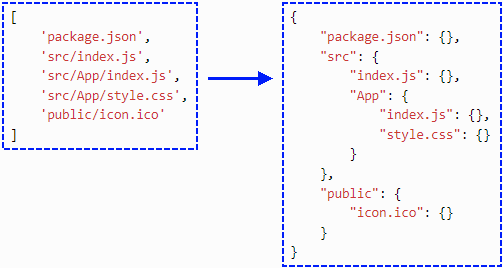
Practical example:
xxxxxxxxxx
1
const SEPARATOR_EXPRESSION = /[\\\/¥₩]+/i;
2
3
const toTree = (paths) => {
4
const tree = {};
5
for (let i = 0; i < paths.length; ++i) {
6
const path = paths[i];
7
if (path) {
8
let node = tree;
9
const parts = path.split(SEPARATOR_EXPRESSION);
10
for (let j = 0; j < parts.length; ++j) {
11
const part = parts[j];
12
if (part) {
13
node = node[part] ?? (node[part] = {});
14
}
15
}
16
}
17
}
18
return tree;
19
};
20
21
22
// Usage example:
23
24
const paths = [
25
'package.json',
26
'src/index.js',
27
'src/App/index.js',
28
'src/App/style.css',
29
'public/icon.ico'
30
];
31
32
const tree = toTree(paths);
33
34
console.log(JSON.stringify(tree, null, 4));
Notes:
¥
and₩
were used because of possible Japanese and Korean path separators,- in practise web browsers' APIs work on slashes only, so it is not needed to use
¥
and₩
separators.
Warning: this solution should be used only with iterable collections.
xxxxxxxxxx
1
const SEPARATOR_EXPRESSION = /[\\\/¥₩]+/i;
2
3
const toTree = (paths) => {
4
const tree = {};
5
for (const path of paths) {
6
if (path) {
7
let node = tree;
8
const parts = path.split(SEPARATOR_EXPRESSION);
9
for (const part of parts) {
10
if (part) {
11
node = node[part] ?? (node[part] = {});
12
}
13
}
14
}
15
}
16
return tree;
17
};
18
19
20
// Usage example:
21
22
const paths = [
23
'package.json',
24
'src/index.js',
25
'src/App/index.js',
26
'src/App/style.css',
27
'public/icon.ico'
28
];
29
30
const tree = toTree(paths);
31
32
console.log(JSON.stringify(tree, null, 4));