EN
JavaScript - beautiful html alerts with Sweet Alert 2 - full code examples
5
points
1. Sweet Alert 2 - success alert example
// ONLINE-RUNNER:browser;
<!doctype html>
<html>
<head>
<script src="https://cdn.jsdelivr.net/npm/sweetalert2@9"></script>
</head>
<body style="font-family: sans-serif; ">
<div style="height: 500px;">
<button onclick="fireSweetAlert()">Show sweet alert example</button>
</div>
<script>
function fireSweetAlert() {
Swal.fire(
'Good job!',
'You clicked the button!',
'success'
)
}
</script>
</body>
</html>
Screenshot with expected results:
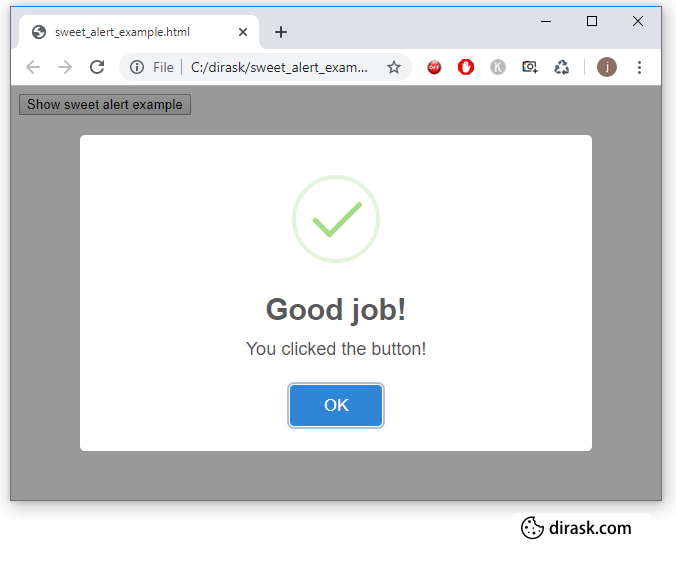
2. Sweet Alert 2 - info message example
// ONLINE-RUNNER:browser;
<!doctype html>
<html>
<head>
<script src="https://cdn.jsdelivr.net/npm/sweetalert2@9"></script>
</head>
<body style="font-family: sans-serif; ">
<div style="height: 500px;">
<button onclick="fireSweetAlert()">Show sweet alert example</button>
</div>
<script>
function fireSweetAlert() {
Swal.fire({
icon: 'info',
title: 'Info',
text: 'You clicked the button!'
})
}
</script>
</body>
</html>
Screenshot with expected results:
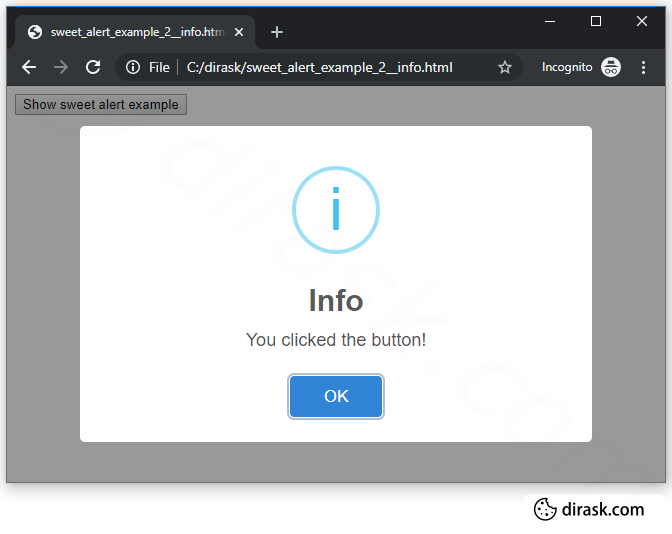
3. Sweet Alert 2 - error message example
// ONLINE-RUNNER:browser;
<!doctype html>
<html>
<head>
<script src="https://cdn.jsdelivr.net/npm/sweetalert2@9"></script>
</head>
<body style="font-family: sans-serif; ">
<div style="height: 500px;">
<button onclick="fireSweetAlert()">Show sweet alert example</button>
</div>
<script>
function fireSweetAlert() {
Swal.fire({
icon: 'error',
title: 'Oops...',
text: 'Something went wrong!'
})
}
</script>
</body>
</html>
Screenshot with expected results:
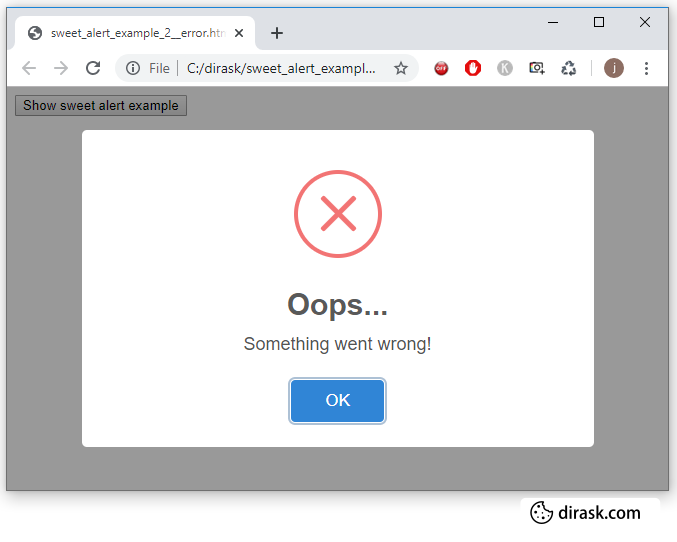
4. Sweet Alert 2 - five examples in one file
// ONLINE-RUNNER:browser;
<!doctype html>
<html>
<head>
<script src="https://cdn.jsdelivr.net/npm/sweetalert2@9"></script>
<style>
.btn {
margin: 10px;
}
</style>
</head>
<body style="font-family: sans-serif; ">
<div style="height: 500px;">
<div class="btn"><button onclick="showSuccessAlert()">Show success</button></div>
<div class="btn"><button onclick="fireInfoAlert()">Show info</button></div>
<div class="btn"><button onclick="showError()">Show error</button></div>
<div class="btn"><button onclick="showMessage()">Show message</button></div>
<div class="btn">
<button onclick="showAlertTopEnd()">
Show alert in top right corner
</button>
</div>
<div class="btn">
<button onclick="showDialogWithPassingParams()">
Show dialog with second alert on yes
</button>
</div>
</div>
<script>
function showSuccessAlert() {
Swal.fire(
'Good job!',
'You clicked the button!',
'success'
)
}
function fireInfoAlert() {
Swal.fire({
icon: 'info',
title: 'Info',
text: 'You clicked the button!'
})
}
function showError() {
Swal.fire({
icon: 'error',
title: 'Oops...',
text: 'Something went wrong!'
})
}
function showMessage() {
Swal.fire('Simple message')
}
function showAlertTopEnd() {
Swal.fire({
position: 'top-end',
icon: 'success',
title: 'Display this alert in top right corner',
showConfirmButton: false,
timer: 2500
})
}
function showDialogWithPassingParams() {
Swal.fire({
title: 'Are you sure?',
text: "You won't be able to revert this!",
icon: 'warning',
showCancelButton: true,
confirmButtonColor: '#3085d6',
cancelButtonColor: '#d33',
confirmButtonText: 'Yes, delete it!'
}).then((result) => {
if (result.value) {
Swal.fire(
'Deleted!',
'Your file has been deleted.',
'success'
)
}
})
}
</script>
</body>
</html>