EN
JavaScript - base64 with Unicode support
9
points
In this short article, we would like to show how to encode text to base64 with Unicode support and back (decode base64) in JavaScript.
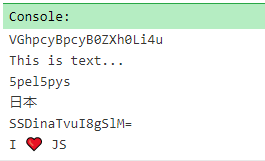
Quick solution (safe approach):
// ONLINE-RUNNER:browser;
var toUtf8 = function(text) {
var surrogate = encodeURIComponent(text);
var result = '';
for (var i = 0; i < surrogate.length;) {
var character = surrogate[i];
i += 1;
if (character == '%') {
var hex = surrogate.substring(i, i += 2);
if (hex) {
result += String.fromCharCode(parseInt(hex, 16));
}
} else {
result += character;
}
}
return result;
};
var fromUtf8 = function(text) {
var result = '';
for (var i = 0; i < text.length; ++i) {
var code = text.charCodeAt(i);
result += '%';
if (code < 16) {
result += '0';
}
result += code.toString(16);
}
return decodeURIComponent(result);
};
var Base64 = {
encode: function(text) {
return btoa(toUtf8(text));
},
decode: function(base64) {
return fromUtf8(atob(base64));
}
};
// Example:
console.log(Base64.encode('This is text...')); // VGhpcyBpcyB0ZXh0Li4u
console.log(Base64.decode('VGhpcyBpcyB0ZXh0Li4u')); // This is text...
console.log(Base64.encode('日本')); // 5pel5pys
console.log(Base64.decode('5pel5pys')); // 日本
console.log(Base64.encode('I ❤️ JS')); // SSDinaTvuI8gSlM=
console.log(Base64.decode('SSDinaTvuI8gSlM=')); // I ❤️ JS
Web browser methods based approach
Note:
escape
andunescape
methods are marked as not recommended to use so read about them here and here.
// ONLINE-RUNNER:browser;
var toUtf8 = function(text) {
return unescape(encodeURIComponent(text));
};
var fromUtf8 = function(text) {
return decodeURIComponent(escape(text));
};
var Base64 = {
encode: function(text) {
return btoa(toUtf8(text));
},
decode: function(base64) {
return fromUtf8(atob(base64));
}
};
// Example:
console.log(Base64.encode('This is text...')); // VGhpcyBpcyB0ZXh0Li4u
console.log(Base64.decode('VGhpcyBpcyB0ZXh0Li4u')); // This is text...
console.log(Base64.encode('日本')); // 5pel5pys
console.log(Base64.decode('5pel5pys')); // 日本
console.log(Base64.encode('I ❤️ JS')); // SSDinaTvuI8gSlM=
console.log(Base64.decode('SSDinaTvuI8gSlM=')); // I ❤️ JS