JavaScript - Math.atan2() method example
The Math.atan2()
function returns the angle in radians in the range -Math.PI/2
to +Math.PI/2
between the positive x-axis and the ray to the point (x, y) ≠ (0, 0).
// ONLINE-RUNNER:browser;
// y x angle in radians
console.log( Math.atan2( 2, 4) ); // 0.4636476090008061 <- ~26.6 degrees
console.log( Math.atan2( 4, -2) ); // 2.0344439357957027 <- ~116.6 degrees
console.log( Math.atan2(-2, -4) ); // -2.6779450445889870 <- ~-153.4 degrees
console.log( Math.atan2(-4, 2) ); // -1.1071487177940904 <- ~-63.4 degrees
atan2()
method has been visualized on below image:
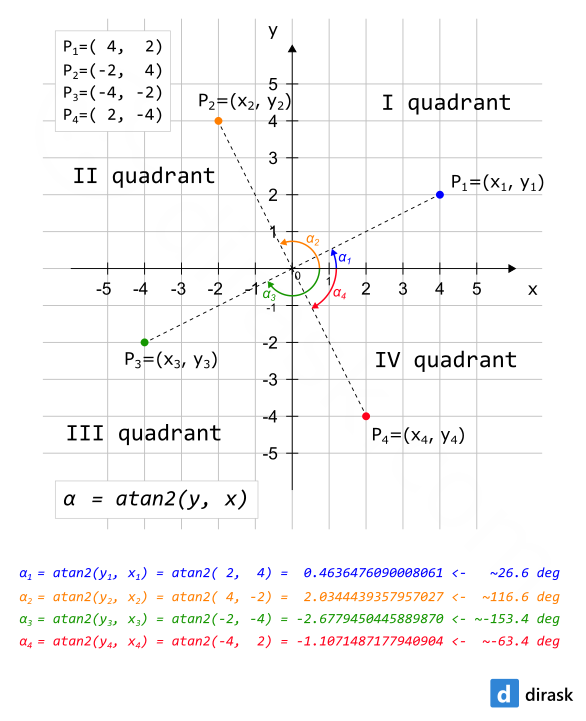
1. Documentation
Syntax | Math.atan2(y, x) |
Parameters | y , x - integer or float number values that are coordinates of point (primitive value). |
Result |
Where:
If point is in the 1st (I) or 2nd (II) quadrant angle is measured in a counterclockwise direction. If point is in the 3rd (III) or 4th (IV) quadrant angle is measured in a clockwise direction.
|
Description |
|
2. Working with degrees
// ONLINE-RUNNER:browser;
function calculateAngle(y, x) {
var angle = Math.atan2(y, x);
return (180 / Math.PI) * angle; // rad to deg conversion
}
// Example usage:
console.log( calculateAngle( 2, 4) ); // 26.56505117707799 degrees
console.log( calculateAngle( 4, -2) ); // 116.56505117707799 degrees
console.log( calculateAngle(-2, -4) ); // -153.43494882292200 degrees
console.log( calculateAngle(-4, 2) ); // -63.43494882292201 degrees
3. Conversion to only clockwise angles in degrees
This section shows how to convert angles to clockwise angles (from 0 to 360 degrees).
// ONLINE-RUNNER:browser;
function calculateAngle(y, x) {
var angle = Math.atan2(y, x);
if (angle < 0.0) {
angle += 2.0 * Math.PI;
}
return (180 / Math.PI) * angle; // rad to deg conversion
}
// Example usage:
console.log( calculateAngle( 2, 4) ); // 26.56505117707799 degrees
console.log( calculateAngle( 4, -2) ); // 116.56505117707799 degrees
console.log( calculateAngle(-2, -4) ); // 206.56505117707800 degrees
console.log( calculateAngle(-4, 2) ); // 296.56505117707800 degrees
4. Conversion to only counterclockwise angles in degrees
This section shows how to convert angles to counterclockwise angles (from -360 to 0 degrees).
// ONLINE-RUNNER:browser;
function calculateAngle(y, x) {
var angle = Math.atan2(y, x);
if (angle > 0.0) {
angle -= 2.0 * Math.PI;
}
return (180 / Math.PI) * angle; // rad to deg conversion
}
// Example usage:
console.log( calculateAngle( 2, 4) ); // -333.434948822922 degrees
console.log( calculateAngle( 4, -2) ); // -243.434948822922 degrees
console.log( calculateAngle(-2, -4) ); // -153.434948822922 degrees
console.log( calculateAngle(-4, 2) ); // -63.4349488229220 degrees