HTML - Element, Text, Comment, Node, etc. inheritance
In this article, we would like to show you HTML elements inheritance concept.
Every HTML element is a Node (it means HTMLElement extends Element that extends Node, etc.). As you can see, Text and Comment are located on different branches and they are also Nodes. Elements like: <div>
, <p>
, <img>
, <br>
, <body>
, etc. are HTMLElements.
Overview:
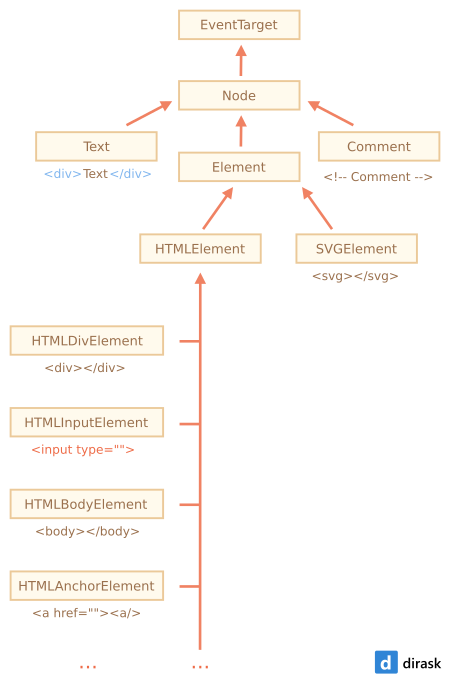
Practical examples
In this section, we create instances of Text, Element, and Comment HTML elements and use instanceof
operator to present the elements inheritance.
Text
Text is a node that presents text on a website. Text nodes are located in HTML elements.
// ONLINE-RUNNER:browser;
// create text node
const textNode = document.createTextNode('text');
// check if the node is instance of Text, Node, etc.
console.log(textNode instanceof Text); // true
console.log(textNode instanceof Node); // true
console.log(textNode instanceof EventTarget); // true
console.log(textNode instanceof Comment); // false
console.log(textNode instanceof Element); // false
console.log(textNode instanceof HTMLElement); // false
HTMLElement
HTML elements build website layout. In the below example we used <div>
as an example element.
// ONLINE-RUNNER:browser;
// create element
const element = document.createElement('div');
// check if the element is instance of Element, Node, etc.
console.log(element instanceof HTMLElement); // true
console.log(element instanceof Element); // true
console.log(element instanceof Node); // true
console.log(element instanceof EventTarget); // true
console.log(element instanceof Text); // false
console.log(element instanceof Comment); // false
Comment
Comments are useful to mark some important things in the source core. The user doesn't see them by default.
// ONLINE-RUNNER:browser;
// create comment
const comment = document.createComment('comment')
// check if the comment is instance of Comment, Node, etc.
console.log(comment instanceof Comment); // true
console.log(comment instanceof Node); // true
console.log(comment instanceof EventTarget); // true
console.log(comment instanceof Text); // false
console.log(comment instanceof Element); // false
console.log(comment instanceof HTMLElement); // false