EN
C# / .NET - Math.Log() method example
0 points
The Math.Log()
method returns the natural logarithm (base e) of a number.
xxxxxxxxxx
1
using System;
2
3
public class Program
4
{
5
public static void Main(string[] args)
6
{
7
// Natural logarithm (logarithm with base e):
8
// x y
9
Console.WriteLine( Math.Log( 1 ) ); // 0
10
Console.WriteLine( Math.Log( 7 ) ); // 1.9459101490553132
11
Console.WriteLine( Math.Log( 10 ) ); // 2.3025850929940460
12
Console.WriteLine( Math.Log( 100 ) ); // 4.6051701859880920
13
Console.WriteLine( Math.Log( 1000 ) ); // 6.9077552789821370
14
15
Console.WriteLine( Math.Log( -1 ) ); // NaN
16
Console.WriteLine( Math.Log( 0 ) ); // -∞ / -Infinity
17
Console.WriteLine( Math.Log( Double.PositiveInfinity ) ); // ∞ / +Infinity
18
19
Console.WriteLine( Math.E ); // 2.718281828459045
20
21
// Logarithm with custom base is placed in the below example.
22
}
23
}
The Math.Log()
method is presented on the following chart:
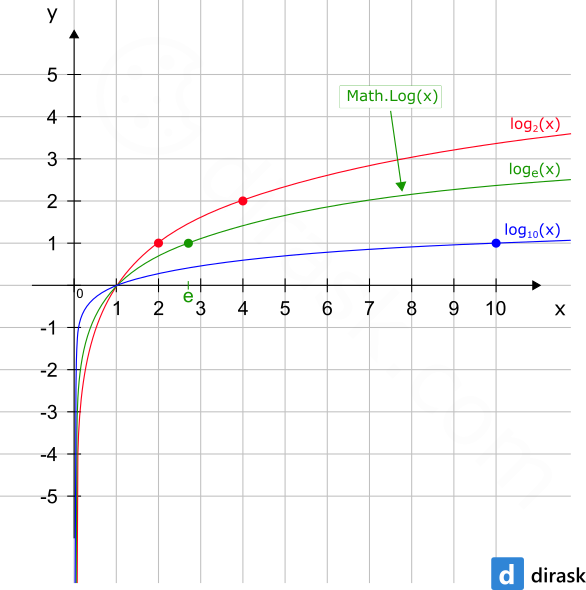
Syntax |
xxxxxxxxxx 1 namespace System 2 { 3 public static class Math 4 { 5 // ... 6 public static double Log(double a, double newBase) { ... } 7 public static double Log(double d) { ... } 8 // ... 9 } 10 } |
Parameters |
|
Result |
If If If |
Description |
|
This example shows a logarithmic function calculation with its own base.
xxxxxxxxxx
1
using System;
2
3
public class Program
4
{
5
static double calculateLogarithm(double logBase, double x)
6
{
7
double a = Math.Log(x);
8
double b = Math.Log(logBase);
9
10
return a / b;
11
}
12
13
public static void Main(string[] args)
14
{
15
// Logarithm with custom base:
16
// base x y
17
Console.WriteLine( calculateLogarithm( 2, 2 ) ); // 1
18
Console.WriteLine( calculateLogarithm( 2, 4 ) ); // 2
19
Console.WriteLine( calculateLogarithm( Math.E, Math.E ) ); // 1
20
Console.WriteLine( calculateLogarithm( 3, 9 ) ); // 2
21
Console.WriteLine( calculateLogarithm( 3, 81 ) ); // 4
22
Console.WriteLine( calculateLogarithm( 10, 10 ) ); // 1
23
}
24
}