C# / .NET - Math.Atan2() method example
The Math.Atan2()
function returns the angle in radians in the range -Math.PI/2
to +Math.PI/2
between the positive x-axis and the ray to the point (x, y) ≠ (0, 0).
using System;
public class Program
{
public static void Main(string[] args)
{
// y x angle in radians
Console.WriteLine(Math.Atan2( 2, 4)); // 0.4636476090008061 <- ~26.6 degrees
Console.WriteLine(Math.Atan2( 4, -2)); // 2.0344439357957027 <- ~116.6 degrees
Console.WriteLine(Math.Atan2(-2, -4)); // -2.677945044588987 <- ~-153.4 degrees
Console.WriteLine(Math.Atan2(-4, 2)); // -1.1071487177940904 <- ~-63.4 degrees
}
}
Atan2()
method has been visualized on below image:
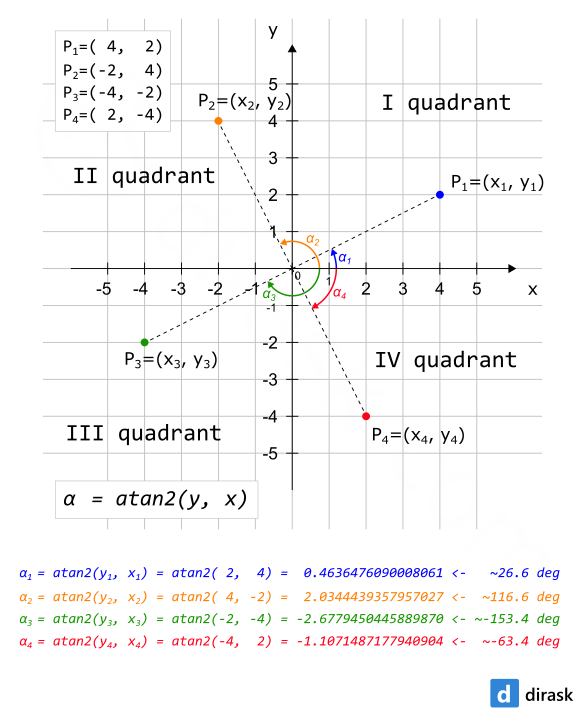
1. Documentation
Syntax |
|
Parameters | y , x - double values that are coordinates of the point (primitive value). |
Result |
Where:
If point is in the 1st (I) or 2nd (II) quadrant angle is measured in a counterclockwise direction. If point is in the 3rd (III) or 4th (IV) quadrant angle is measured in a clockwise direction.
|
Description |
|
2. Working with degrees
using System;
public class Program
{
static double CalculateAngle(double y, double x)
{
double angle = Math.Atan2(y, x);
return (180 / Math.PI) * angle; // rad to deg conversion
}
public static void Main(string[] args)
{
// y x degrees
Console.WriteLine( CalculateAngle( 2, 4)); // 26.56505117707799
Console.WriteLine( CalculateAngle( 4, -2)); // 116.56505117707799
Console.WriteLine( CalculateAngle(-2, -4)); // -153.434948822922
Console.WriteLine( CalculateAngle(-4, 2)); // -63.43494882292201
}
}
3. Conversion to only clockwise angles in degrees
This section shows how to convert angles to clockwise angles (from 0 to 360 degrees).
using System;
public class Program
{
static double CalculateAngle(double y, double x)
{
double angle = Math.Atan2(y, x);
if (angle < 0.0)
{
angle += 2.0 * Math.PI;
}
return (180 / Math.PI) * angle; // rad to deg conversion
}
public static void Main(string[] args)
{
// y x degrees
Console.WriteLine( CalculateAngle( 2, 4)); // 26.56505117707799
Console.WriteLine( CalculateAngle( 4, -2)); // 116.56505117707799
Console.WriteLine( CalculateAngle(-2, -4)); // 206.565051177078
Console.WriteLine( CalculateAngle(-4, 2)); // 296.565051177078
}
}
4. Conversion to only counterclockwise angles in degrees
This section shows how to convert angles to counterclockwise angles (from -360 to 0 degrees).
using System;
public class Program
{
static double CalculateAngle(double y, double x)
{
double angle = Math.Atan2(y, x);
if (angle > 0.0)
{
angle -= 2.0 * Math.PI;
}
return (180 / Math.PI) * angle; // rad to deg conversion
}
public static void Main(string[] args)
{
// y x degrees
Console.WriteLine( CalculateAngle( 2, 4)); // -333.434948822922
Console.WriteLine( CalculateAngle( 4, -2)); // -243.434948822922
Console.WriteLine( CalculateAngle(-2, -4)); // -153.434948822922
Console.WriteLine( CalculateAngle(-4, 2)); // -63.43494882292201
}
}